A circular linked list is a type of linked list where the last node points back to the first node, forming a circle. This structure is particularly useful in scenarios where continuous traversal of elements is required, such as implementing buffers, job scheduling, and more. One common operation on a circular linked list is deleting the first node, a task that requires careful handling to maintain the list’s circular nature.
Table of Contents
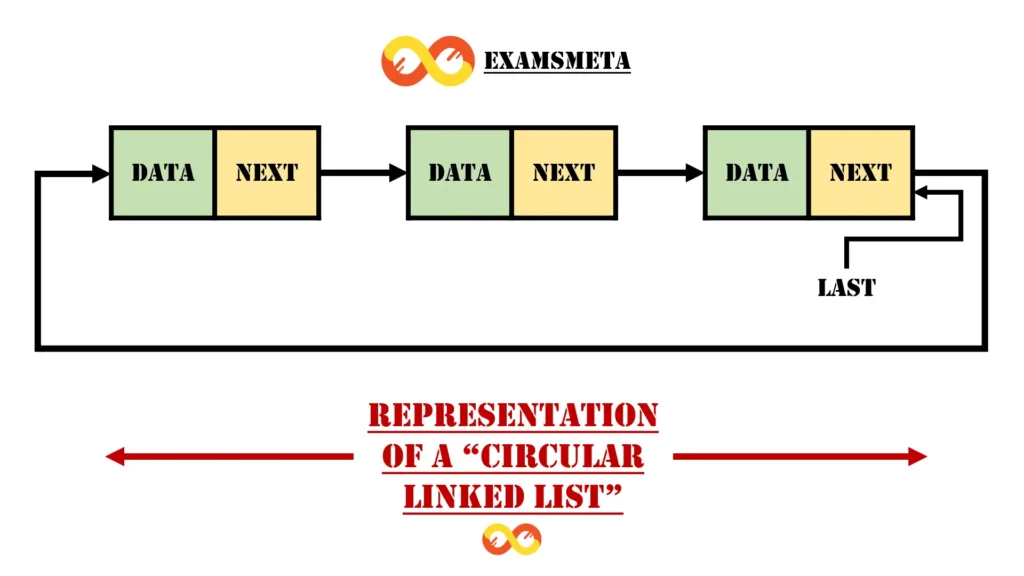
In this detailed post, we will explore the process of deleting the first node from a circular linked list (Deletion from the Beginning of a Circular Linked List). We’ll delve into a step-by-step explanation, present a C programming and multiple programming languages implementation, and examine the time and space complexities. Let’s start by understanding the logic behind this operation.
Informative Table
Here’s a detailed table summarizing the key points from the article:
Aspect | Details |
---|---|
Data Structure | Circular Linked List |
Operation | Deletion of the first node |
Key Cases | – Empty List: No nodes to delete- Single Node: Deleting the only node makes the list empty- Multiple Nodes: Adjust pointers to maintain the circular structure |
Steps to Delete | 1. Check if Empty: If last == NULL , print “List is empty” and return NULL .2. Retrieve Head: Set head = last->next .3. Check Single Node: If head == last , free head and set last = NULL .4. Handle Multiple Nodes: Update last->next to head->next and free head .5. Return Updated Last. |
Code Implementation | Written in C programming and other multiple programming languages, including functions for:- Deleting the first node- Printing the list- Creating new nodes. |
Output Example | – Before Deletion: 2 3 4 – After Deletion: 3 4 |
Time Complexity | O(1) – Deletion involves a fixed number of operations regardless of the list size. |
Space Complexity | O(1) – No additional data structures are used. |
Edge Cases | – Empty list: Return NULL .- Single node: Free the node and set last = NULL .- Multiple nodes: Ensure the circular structure is maintained. |
Use Cases | – Round-robin scheduling– Music playlists– FIFO buffers |
Key Functions in Code | – deleteFirstNode : Deletes the first node and updates the list.- printList : Prints the circular linked list.- createNode : Creates a new node with given data. |
Advantages | – Maintains circular structure after deletion.- Efficient constant-time operation for deleting the first node. |
Limitations | – Requires proper handling of edge cases (empty and single-node lists). |
Programming Language | C programming language and other multiple programming languages |
This table captures all the essential details in a structured and easily digestible format.
Understanding the Deletion Process
When deleting the first node in a circular linked list, several cases must be considered:
- Empty List: If the list is empty, there are no nodes to delete.
- Single Node: If the list contains only one node, deleting it will result in an empty list.
- Multiple Nodes: For a list with multiple nodes, special care is required to ensure that the circular structure remains intact.
Each of these cases is handled in a specific manner, ensuring that the list remains functional after the operation.
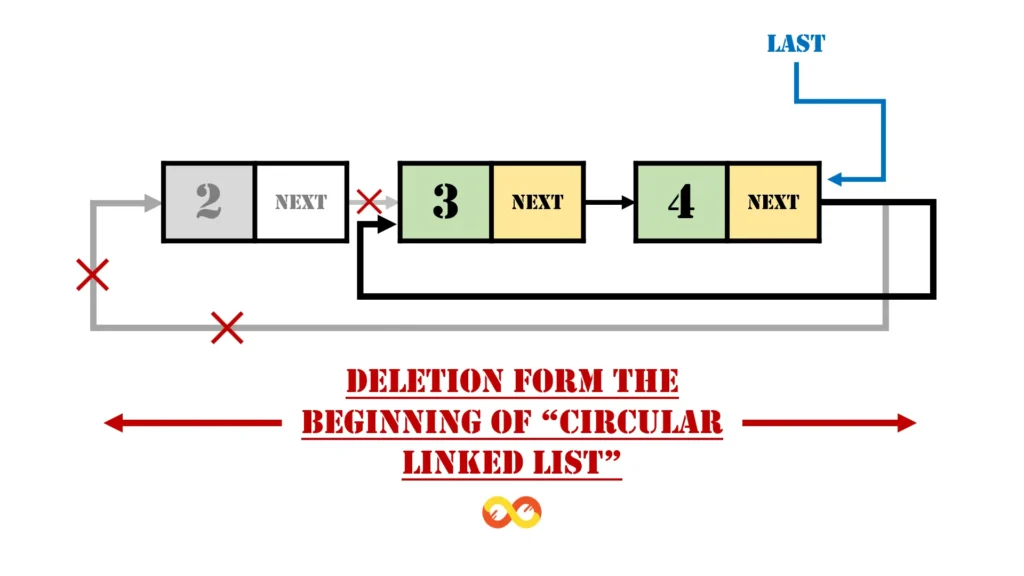
Step-by-Step Approach
Here’s a detailed breakdown of the steps involved in deleting the first node of a circular linked list:
1. Check if the List is Empty
The first step is to determine if the list is empty. In a circular linked list, an empty list is represented by a nullptr
(or NULL
in C). If the list is empty:
- Print an appropriate message, e.g., “List is empty.”
- Return
nullptr
, as no further operations are required.
2. Retrieve the Head Node
The head node in a circular linked list is the node immediately following the last node. This is accessed using the pointer last->next
.
3. Check if the List Contains a Single Node
If the head node is the same as the last node:
- Free the memory allocated for the head node using
free(head)
in C. - Set the
last
pointer tonullptr
, signifying an empty list.
4. Handle Multiple Nodes
If the list contains more than one node:
- Update the
last->next
pointer to bypass the current head node, effectively removing it from the list. - Free the memory allocated for the removed head node.
5. Return the Updated Last Pointer
After completing the above steps, return the updated last
pointer, which still points to the last node in the modified list.
Implementation in C Programming
The following C code demonstrates the process of deleting the first node from a circular linked list. It includes helper functions for creating nodes, printing the list, and performing the deletion operation.
#include <stdio.h>
#include <stdlib.h>
// Define the structure of a Node
struct Node {
int data;
struct Node* next;
};
// Function to delete the first node of a circular linked list
struct Node* deleteFirstNode(struct Node* last) {
if (last == NULL) {
// If the list is empty
printf("List is empty\n");
return NULL;
}
struct Node* head = last->next;
if (head == last) {
// If there is only one node in the list
free(head);
last = NULL;
} else {
// More than one node in the list
last->next = head->next;
free(head);
}
return last;
}
// Function to print the elements of a circular linked list
void printList(struct Node* last) {
if (last == NULL) return;
struct Node* head = last->next;
while (1) {
printf("%d ", head->data);
head = head->next;
if (head == last->next) break;
}
printf("\n");
}
// Function to create a new node
struct Node* createNode(int value) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = value;
newNode->next = NULL;
return newNode;
}
// Main function to demonstrate the operation
int main() {
// Create nodes and link them in a circular fashion
struct Node* first = createNode(2);
first->next = createNode(3);
first->next->next = createNode(4);
struct Node* last = first->next->next;
last->next = first;
// Print the original list
printf("Original list: ");
printList(last);
// Delete the first node
last = deleteFirstNode(last);
// Print the modified list
printf("List after deleting first node: ");
printList(last);
return 0;
}
Output of the Program
Original list: 2 3 4
List after deleting first node: 3 4
Step-by-Step Explanation
- Define the Node Structure
- Each node has two fields:
data
: Stores the integer value of the node.next
: Points to the next node in the circular linked list.
- Each node has two fields:
- Delete the First Node
- The
deleteFirstNode
function handles three cases:- Empty List:
- If the list is empty (
last == NULL
), a message is printed, and the function returnsNULL
.
- If the list is empty (
- Single Node:
- If the list has only one node (
last == last->next
), that node is freed, and the list becomes empty.
- If the list has only one node (
- Multiple Nodes:
- Updates the
next
pointer of the last node to point to the second node. - Frees the memory allocated for the first node.
- Updates the
- Empty List:
- The
- Print the Circular Linked List
- The
printList
function:- If the list is empty, prints “List is empty.”
- Otherwise, starts from the first node and traverses the list in a circular manner until it loops back to the starting node.
- The
- Create Nodes
- The
createNode
function:- Allocates memory for a new node.
- Initializes its
data
with the given value and sets itsnext
toNULL
.
- The
- Main Function
- Demonstrates the functionality:
- Step 1: Creates a circular linked list with three nodes (
2 -> 3 -> 4
). - Step 2: Prints the original list.
- Step 3: Deletes the first node using
deleteFirstNode
. - Step 4: Prints the list after the deletion.
- Step 1: Creates a circular linked list with three nodes (
- Demonstrates the functionality:
Explanation of the Output
- The original circular linked list (
2 -> 3 -> 4
) is displayed correctly. - After deleting the first node, the list becomes (
3 -> 4
) with3
as the new head. The circular structure is maintained.
Code Implementation In Multiple Programming Languages
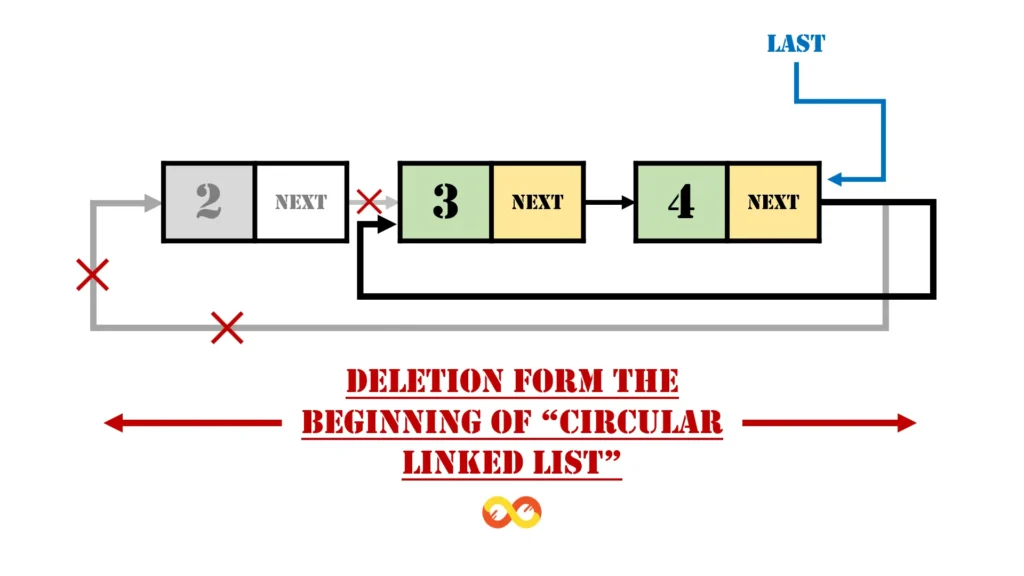
C Programming
#include <stdio.h>
#include <stdlib.h>
// Define the structure of a node
struct Node {
int data;
struct Node* next;
};
// Function to delete the first node in the circular linked list
struct Node* deleteFirstNode(struct Node* last) {
if (last == NULL) {
printf("List is empty\n");
return NULL;
}
struct Node* head = last->next;
if (head == last) {
free(head);
return NULL;
}
last->next = head->next;
free(head);
return last;
}
// Function to print the circular linked list
void printList(struct Node* last) {
if (last == NULL) {
printf("List is empty.\n");
return;
}
struct Node* head = last->next;
do {
printf("%d ", head->data);
head = head->next;
} while (head != last->next);
printf("\n");
}
// Function to create a new node with the given value
struct Node* createNode(int value) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = value;
newNode->next = NULL;
return newNode;
}
// Main function
int main() {
struct Node* first = createNode(2);
first->next = createNode(3);
first->next->next = createNode(4);
struct Node* last = first->next->next;
last->next = first;
printf("Original list: ");
printList(last);
last = deleteFirstNode(last);
printf("List after deleting first node: ");
printList(last);
return 0;
}
C++ Implementation
#include <iostream>
using namespace std;
// Node structure
struct Node {
int data;
Node* next;
};
// Function to delete the first node
Node* deleteFirstNode(Node* last) {
if (!last) {
cout << "List is empty" << endl;
return nullptr;
}
Node* head = last->next;
if (head == last) {
delete head;
return nullptr;
}
last->next = head->next;
delete head;
return last;
}
// Function to print the list
void printList(Node* last) {
if (!last) {
cout << "List is empty." << endl;
return;
}
Node* head = last->next;
do {
cout << head->data << " ";
head = head->next;
} while (head != last->next);
cout << endl;
}
// Function to create a new node
Node* createNode(int value) {
Node* newNode = new Node();
newNode->data = value;
newNode->next = nullptr;
return newNode;
}
// Main function
int main() {
Node* first = createNode(2);
first->next = createNode(3);
first->next->next = createNode(4);
Node* last = first->next->next;
last->next = first;
cout << "Original list: ";
printList(last);
last = deleteFirstNode(last);
cout << "List after deleting first node: ";
printList(last);
return 0;
}
C# Implementation
using System;
class Node {
public int Data;
public Node Next;
public Node(int data) {
Data = data;
Next = null;
}
}
class CircularLinkedList {
public Node Last;
public void DeleteFirstNode() {
if (Last == null) {
Console.WriteLine("List is empty");
return;
}
Node head = Last.Next;
if (head == Last) {
Last = null;
return;
}
Last.Next = head.Next;
}
public void PrintList() {
if (Last == null) {
Console.WriteLine("List is empty.");
return;
}
Node head = Last.Next;
do {
Console.Write(head.Data + " ");
head = head.Next;
} while (head != Last.Next);
Console.WriteLine();
}
public void CreateNode(int[] values) {
foreach (var value in values) {
Node newNode = new Node(value);
if (Last == null) {
Last = newNode;
Last.Next = Last;
} else {
newNode.Next = Last.Next;
Last.Next = newNode;
Last = newNode;
}
}
}
}
class Program {
static void Main() {
CircularLinkedList list = new CircularLinkedList();
list.CreateNode(new int[] { 2, 3, 4 });
Console.WriteLine("Original list: ");
list.PrintList();
list.DeleteFirstNode();
Console.WriteLine("List after deleting first node: ");
list.PrintList();
}
}
Java Implementation
class Node {
int data;
Node next;
Node(int data) {
this.data = data;
this.next = null;
}
}
public class CircularLinkedList {
Node last;
// Delete the first node
public void deleteFirstNode() {
if (last == null) {
System.out.println("List is empty");
return;
}
Node head = last.next;
if (head == last) {
last = null;
return;
}
last.next = head.next;
}
// Print the circular linked list
public void printList() {
if (last == null) {
System.out.println("List is empty.");
return;
}
Node head = last.next;
do {
System.out.print(head.data + " ");
head = head.next;
} while (head != last.next);
System.out.println();
}
// Create a circular linked list from an array of values
public void createList(int[] values) {
for (int value : values) {
Node newNode = new Node(value);
if (last == null) {
last = newNode;
last.next = last;
} else {
newNode.next = last.next;
last.next = newNode;
last = newNode;
}
}
}
public static void main(String[] args) {
CircularLinkedList list = new CircularLinkedList();
list.createList(new int[] { 2, 3, 4 });
System.out.println("Original list:");
list.printList();
list.deleteFirstNode();
System.out.println("List after deleting first node:");
list.printList();
}
}
- Step-by-Step Explanation
- Node Definition: A
Node
class is defined with two fields:data
for storing the value andnext
for pointing to the next node. - CircularLinkedList Class:
last
points to the last node in the circular linked list.- Methods like
deleteFirstNode()
,printList()
, andcreateList()
are provided to manage the list.
- createList(): Creates the circular linked list from an array of integers.
- deleteFirstNode():
- Handles the cases where the list is empty, has only one node, or has multiple nodes.
- Updates the
last
pointer to maintain the circular structure after deletion.
- printList(): Prints all nodes in the list starting from the node after
last
. - Main Method: Creates the list, prints it, deletes the first node, and prints the updated list.
- Node Definition: A
JavaScript Implementation
class Node {
constructor(data) {
this.data = data;
this.next = null;
}
}
class CircularLinkedList {
constructor() {
this.last = null;
}
deleteFirstNode() {
if (this.last === null) {
console.log("List is empty");
return;
}
let head = this.last.next;
if (head === this.last) {
this.last = null;
return;
}
this.last.next = head.next;
}
printList() {
if (this.last === null) {
console.log("List is empty.");
return;
}
let head = this.last.next;
let result = [];
do {
result.push(head.data);
head = head.next;
} while (head !== this.last.next);
console.log(result.join(" "));
}
createList(values) {
values.forEach(value => {
const newNode = new Node(value);
if (this.last === null) {
this.last = newNode;
this.last.next = this.last;
} else {
newNode.next = this.last.next;
this.last.next = newNode;
this.last = newNode;
}
});
}
}
// Main program
const list = new CircularLinkedList();
list.createList([2, 3, 4]);
console.log("Original list:");
list.printList();
list.deleteFirstNode();
console.log("List after deleting first node:");
list.printList();
- Step-by-Step Explanation
- Node Class: A
Node
class is defined with propertiesdata
(to store value) andnext
(to store the reference to the next node). - CircularLinkedList Class:
last
points to the last node.- Methods include
deleteFirstNode()
,printList()
, andcreateList()
.
- createList(): Iterates through the given array to build the circular linked list.
- deleteFirstNode():
- Checks if the list is empty.
- If there’s only one node, sets
last
tonull
. - Otherwise, adjusts the
last.next
pointer to bypass the first node.
- printList(): Uses a loop to traverse and print all node values.
- Main Program: Creates a list, prints it, deletes the first node, and prints the updated list.
- Node Class: A
Python Implementation
class Node:
def __init__(self, data):
self.data = data
self.next = None
class CircularLinkedList:
def __init__(self):
self.last = None
def delete_first_node(self):
if self.last is None:
print("List is empty")
return
head = self.last.next
if head == self.last:
self.last = None
return
self.last.next = head.next
def print_list(self):
if self.last is None:
print("List is empty.")
return
head = self.last.next
result = []
while True:
result.append(head.data)
head = head.next
if head == self.last.next:
break
print(" ".join(map(str, result)))
def create_list(self, values):
for value in values:
new_node = Node(value)
if self.last is None:
self.last = new_node
self.last.next = self.last
else:
new_node.next = self.last.next
self.last.next = new_node
self.last = new_node
# Main program
list = CircularLinkedList()
list.create_list([2, 3, 4])
print("Original list:")
list.print_list()
list.delete_first_node()
print("List after deleting first node:")
list.print_list()
- Step-by-Step Explanation
- Node Class: Defines a node with
data
(value) andnext
(reference). - CircularLinkedList Class:
last
stores the reference to the last node.delete_first_node()
handles deletion while maintaining the circular structure.
- create_list(): Constructs the list from an array of values.
- delete_first_node(): Deletes the first node while ensuring circularity.
- print_list(): Traverses and prints the list in a circular manner.
- Main Program: Creates, displays, deletes, and re-displays the list.
- Node Class: Defines a node with
Output
Original list:
2 3 4
List after deleting first node:
3 4
Analysis of the Implementation
Time Complexity
The operation of deleting the first node is performed in constant time, as it involves a fixed number of steps:
- Checking if the list is empty.
- Accessing the head node.
- Updating the
last->next
pointer.
Thus, the time complexity of this operation is O(1).
Auxiliary Space
The implementation does not use any additional data structures, and memory is only allocated or freed for nodes in the list. Therefore, the auxiliary space complexity is O(1).
Conclusion
Deleting the first node from a circular linked list is an efficient operation with a constant time complexity. By handling the edge cases (empty list and single-node list) and ensuring that the circular structure remains intact, we can perform this operation safely.
Understanding this process is crucial for mastering circular linked lists, which are widely used in applications such as round-robin scheduling, music playlists, and FIFO buffers. The presented implementation in C programming serves as a foundation for similar operations in other programming languages.
Circular linked lists are a fascinating data structure, and mastering their operations is a step forward in becoming proficient in data structure and algorithm design!
Related Articles
- Introduction to Circular Linked Lists: A Comprehensive Guide
- Understanding Circular Singly Linked Lists: A Comprehensive Guide
- Circular Doubly Linked List: A Comprehensive Guide
- Insertion in Circular Singly Linked List: A Comprehensive Guide
- Insertion in an Empty Circular Linked List: A Detailed Exploration
- Insertion at the Beginning in Circular Linked List: A Detailed Exploration
- Insertion at the End of a Circular Linked List: A Comprehensive Guide
- Insertion at a Specific Position in a Circular Linked List: A Detailed Exploration
- Deletion from a Circular Linked List: A Comprehensive Guide
- Deletion from the Beginning of a Circular Linked List: A Detailed Exploration
- Deletion at Specific Position in Circular Linked List: A Detailed Exploration
- Deletion at the End of a Circular Linked List: A Comprehensive Guide
- Searching in a Circular Linked List: A Comprehensive Exploration
Read More Articles
- Data Structure (DS) Array:
- Why the Analysis of Algorithms is Important?
- Worst, Average, and Best Case Analysis of Algorithms: A Comprehensive Guide
- Understanding Pointers in C Programming: A Comprehensive Guide
- Understanding Arrays in Data Structures: A Comprehensive Exploration
- Memory Allocation of an Array: An In-Depth Comprehensive Exploration
- Understanding Basic Operations in Arrays: A Comprehensive Guide
- Understanding 2D Arrays in Programming: A Comprehensive Guide
- Mapping a 2D Array to a 1D Array: A Comprehensive Exploration
- Data Structure Linked List:
- Understanding Linked Lists in Data Structures: A Comprehensive Exploration
- Types of Linked List: Detailed Exploration, Representations, and Implementations
- Understanding Singly Linked Lists: A Detailed Exploration
- Understanding Doubly Linked List: A Comprehensive Guide
- Operations of Doubly Linked List with Implementation: A Detailed Exploration
- Insertion in Doubly Linked List with Implementation: A Detailed Exploration
- Inserting a Node at the beginning of a Doubly Linked List: A Detailed Exploration
- Inserting a Node After a Given Node in a Doubly Linked List: A Detailed Exploration
- Inserting a Node Before a Given Node in a Doubly Linked List: A Detailed Exploration
- Inserting a Node at a Specific Position in a Doubly Linked List: A Detailed Exploration
- Inserting a New Node at the End of a Doubly Linked List: A Detailed Exploration
- Deletion in a Doubly Linked List with Implementation: A Comprehensive Guide
- Deletion at the Beginning in a Doubly Linked List: A Detailed Exploration
- Deletion after a given node in Doubly Linked List: A Comprehensive Guide
- Deleting a Node Before a Given Node in a Doubly Linked List: A Detailed Exploration
- Deletion at a Specific Position in a Doubly Linked List: A Detailed Exploration
- Deletion at the End in Doubly Linked List: A Comprehensive Exploration
- Introduction to Circular Linked Lists: A Comprehensive Guide
- Understanding Circular Singly Linked Lists: A Comprehensive Guide
- Circular Doubly Linked List: A Comprehensive Guide
- Insertion in Circular Singly Linked List: A Comprehensive Guide
- Insertion in an Empty Circular Linked List: A Detailed Exploration
- Insertion at the Beginning in Circular Linked List: A Detailed Exploration
- Insertion at the End of a Circular Linked List: A Comprehensive Guide
- Insertion at a Specific Position in a Circular Linked List: A Detailed Exploration
- Deletion from a Circular Linked List: A Comprehensive Guide
- Deletion from the Beginning of a Circular Linked List: A Detailed Exploration
- Deletion at Specific Position in Circular Linked List: A Detailed Exploration
- Deletion at the End of a Circular Linked List: A Comprehensive Guide
- Searching in a Circular Linked List: A Comprehensive Exploration
Frequently Asked Questions (FAQs)
What is a Circular Linked List, and how does it differ from a Singly Linked List?
A circular linked list is a variation of the linked list where the last node points back to the first node, forming a closed loop. This design eliminates the nullptr
commonly found at the end of a standard singly linked list, creating a seamless circular structure.
In a singly linked list, traversal stops when the next
pointer of the last node points to nullptr
. However, in a circular linked list, traversal can continue indefinitely as there is no termination point. This structure has significant advantages in scenarios requiring cyclic operations, such as task scheduling, resource allocation, and gaming applications.
The primary distinction lies in the circular nature:
- Singly Linked List: Last node’s
next
pointer isnullptr
. - Circular Linked List: Last node’s
next
pointer points back to the first node.
Why is Deletion from the Beginning of a Circular Linked List Important?
Deleting the first node in a circular linked list is a critical operation used to manage and manipulate the structure efficiently. It is particularly important because:
- The head node (first node) often acts as an entry point for traversal and other operations.
- Removing the head node requires updating the
last->next
pointer to maintain the circular structure, which is unique to this data structure. - Operations like queue management, round-robin scheduling, and memory buffers often involve deletion from the beginning to remove the oldest or least-priority item.
By mastering this operation, programmers can effectively manage dynamic data structures and optimize resource utilization.
What Are the Common Scenarios When Deleting the First Node?
Deleting the first node can occur under three primary scenarios:
- Empty List:
- If the list is empty (i.e.,
last == nullptr
), there is no node to delete. Attempting deletion in such a scenario should gracefully handle the error by checking iflast
isnullptr
before proceeding.
- If the list is empty (i.e.,
- Single Node:
- If the list contains only one node, the
head
andlast
pointers both point to the same node. Deleting this node leaves the list empty, solast
must be updated tonullptr
.
- If the list contains only one node, the
- Multiple Nodes:
- For a list with multiple nodes, the
last->next
pointer must be updated to bypass the currenthead
node, effectively removing it from the list while maintaining the circular structure.
- For a list with multiple nodes, the
What Are the Steps to Delete the First Node from a Circular Linked List?
The process involves the following steps:
- Check if the List is Empty:
- Verify if the
last
pointer isnullptr
. If true, print a message like “List is empty” and return.
- Verify if the
- Retrieve the Head Node:
- Set
head = last->next
to identify the first node in the list.
- Set
- Handle the Single Node Case:
- If
head == last
, delete thehead
node and updatelast = nullptr
to signify an empty list.
- If
- Handle the Multiple Nodes Case:
- Update
last->next
to point tohead->next
, effectively bypassing the current head node. Then, delete thehead
node to free memory.
- Update
- Return the Updated Last Pointer:
- Return the
last
pointer, which remains unchanged in position but points to the updated structure.
- Return the
Why is Maintaining the Circular Structure Important?
The defining feature of a circular linked list is its closed-loop nature. If the circular structure is not maintained during deletion, the list may lose its unique properties, leading to:
- Errors during traversal, as nodes may not connect back to the beginning.
- Inconsistent behavior in applications relying on cyclic operations.
- Potential memory access violations if unreferenced pointers are accessed.
By updating the last->next
pointer during deletion, we ensure that the list remains circular, preventing these issues.
How Do You Handle Memory Management During Node Deletion?
In languages like C++, where memory management is manual, proper deallocation of deleted nodes is crucial to avoid memory leaks. When deleting the head
node:
- Use the
delete
operator to free the allocated memory. - Ensure no dangling pointers reference the deleted node.
For garbage-collected languages like Java or Python, the node becomes eligible for garbage collection once it is removed from the list, provided no external references exist.
What Is the Time Complexity of Deleting the First Node?
The deletion of the first node in a circular linked list has a time complexity of O(1)O(1), making it highly efficient. This efficiency arises because:
- The operation involves direct pointer updates (
last->next
andhead->next
). - No traversal of the list is required, as the
head
andlast
pointers provide direct access to the relevant nodes.
Can Deleting the First Node Lead to Errors?
Errors during deletion typically arise due to:
- Failure to Check for an Empty List:
- Attempting to delete a node when
last == nullptr
can lead to null pointer dereferencing.
- Attempting to delete a node when
- Incorrect Pointer Updates:
- If the
last->next
pointer is not updated correctly, the circular structure is broken, potentially causing traversal loops or memory access issues.
- If the
- Memory Mismanagement:
- Forgetting to delete the
head
node after bypassing it may result in a memory leak.
- Forgetting to delete the
By following a systematic approach and ensuring proper validation, these errors can be mitigated.
What Are the Applications of Circular Linked Lists in Real-World Scenarios?
Circular linked lists are widely used in various applications where cyclic operations are essential:
- Round-Robin Scheduling: In operating systems, circular linked lists manage tasks in a round-robin fashion, ensuring fairness.
- Buffers: Circular buffers use this structure to manage data in systems requiring continuous read/write operations, such as multimedia applications.
- Gaming Applications: Player turns in board games or card games are often managed using circular structures.
- Resource Allocation: Resources like printers or network connections can be distributed in a cyclic manner.
How Can Beginners Practice Deletion in Circular Linked Lists?
To master deletion operations in circular linked lists, beginners can:
- Understand the Theory: Study the structure and properties of circular linked lists, focusing on pointer management and circular integrity.
- Implement Code: Start with basic implementations in C++, Java, Python, or other programming languages.
- Handle Edge Cases: Test scenarios such as empty lists, single-node lists, and multi-node lists to ensure robustness.
- Debug with Diagrams: Visualize the circular structure using diagrams, particularly during pointer updates, to identify and fix errors.
- Explore Applications: Build small projects or simulations like task schedulers or circular buffers to apply the concepts practically.