A Doubly Linked List (DLL) is a type of linked list where each node contains a data part and two pointers: one pointing to the next node and the other pointing to the previous node. This dual linkage makes DLL a versatile data structure for various operations, including deletion, as it allows traversal in both forward and backward directions.
In this guide, we will cover all aspects of deleting nodes in a Doubly Linked List—from deletion at the beginning, at the end, after or before a specific node, and even at a specific position. Each method is explained in detail to ensure clarity and understanding.
Table of Contents
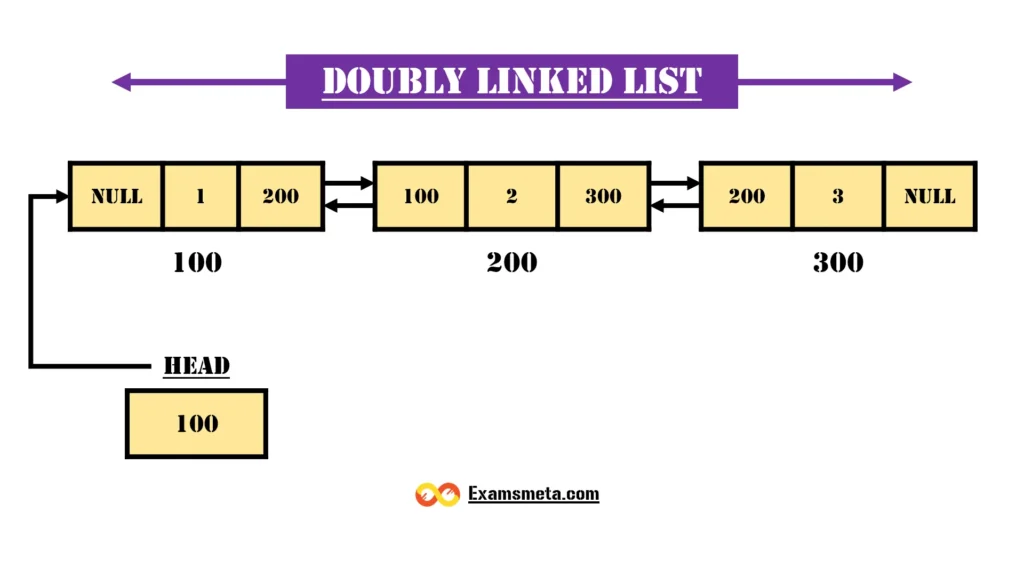
Deletion in a Doubly Linked List
When deleting a node in a Doubly Linked List, special care is needed to ensure that the links between the previous and next nodes are properly maintained. This involves updating the next
pointer of the previous node and the prev
pointer of the next node to bypass the node to be deleted. Let’s delve into each type of deletion:
1. Deletion at the Beginning in a Doubly Linked List
Deleting a node at the beginning is straightforward but requires careful adjustments to maintain the integrity of the list.
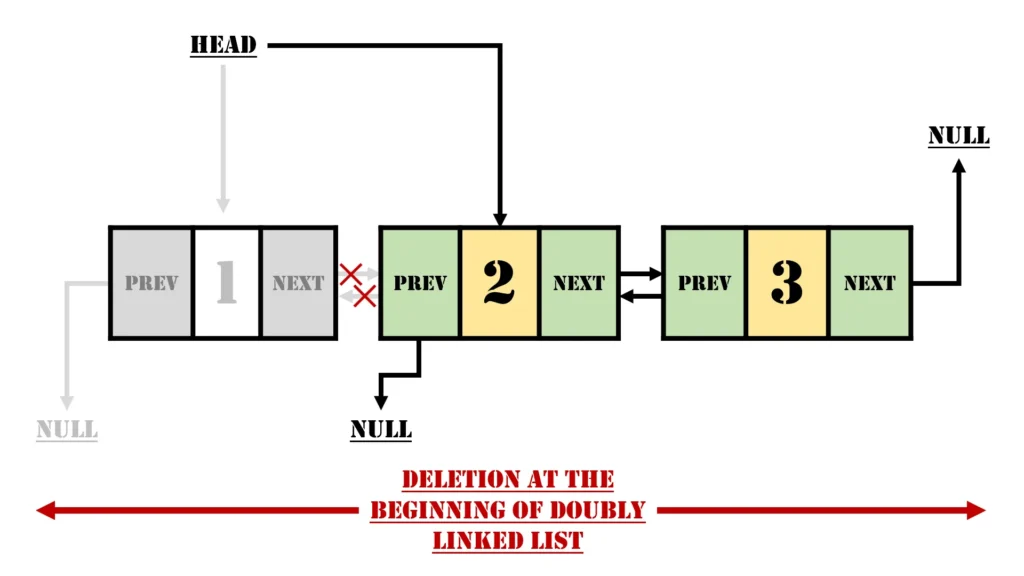
Steps:
- Check if the list is empty: If
head
isNULL
, there’s no node to delete. Exit the function. - Store the current head: Use a temporary pointer, say
temp
, to store the currenthead
node for reference. - Update the head pointer: Set
head
to the next node usinghead = head->next
. - Adjust the new head: If the new
head
is notNULL
, update itsprev
pointer toNULL
usinghead->prev = NULL
. - Free memory: Delete the old head (
temp
) to release allocated memory.
Example:
Input: DLL = 1 <-> 2 <-> 3
Output after deleting the first node: 2 <-> 3
Code Implementation In Python Programming Language
class Node:
def __init__(self, data):
"""Initializes a node with data, prev, and next pointers."""
self.data = data
self.prev = None
self.next = None
def del_head(head):
"""
Deletes the first node (head) of the doubly linked list.
Returns the updated head node.
"""
# Step 1: Check if the list is empty
if head is None:
print("The list is already empty!")
return None
# Step 2: Temporarily store the current head for deletion later
temp = head
# Step 3: Move the head pointer to the next node
head = head.next
# Step 4: Update the prev pointer of the new head, if it exists
if head is not None:
head.prev = None
# Step 5: Delete the old head node (temp)
del temp
# Step 6: Return the updated head pointer
return head
def print_list(head):
"""
Traverses the doubly linked list and prints the data of each node.
"""
# Step 1: Start from the head and move through the list
curr = head
while curr is not None:
print(curr.data, end=" ")
curr = curr.next
print() # Print a new line for cleaner output
if __name__ == "__main__":
"""
Main driver function to demonstrate deleting the head node.
"""
# Step 1: Create a hardcoded doubly linked list
# The list will look like this: 1 <-> 2 <-> 3
head = Node(1) # First node with data = 1
head.next = Node(2) # Second node with data = 2
head.next.prev = head # Link the second node back to the first node
head.next.next = Node(3) # Third node with data = 3
head.next.next.prev = head.next # Link the third node back to the second node
# Step 2: Print the original list
print("Original Linked List:", end=" ")
print_list(head)
# Step 3: Delete the first node (head)
head = del_head(head)
# Step 4: Print the updated list after deletion
print("Deleting Node with data 1 at the Beginning:", end=" ")
print_list(head)
Output:
Original Linked List:
1 2 3
Deleting Node with data 1 at the Beginning:
2 3
Time Complexity: O(1), Since traversal of the linked list is not required.
Auxiliary Space: O(1)
Step-by-Step Explanation of the Code
- Node Class Definition:
- The
Node
class defines the structure of each node in the doubly linked list. - Each node has three attributes:
data
: The value stored in the node.prev
: A pointer to the previous node.next
: A pointer to the next node.
- The
- del_head Function:
- Purpose: Deletes the first node in the list and updates the
head
pointer. - Steps:
- If the list is empty (
head == None
), it prints a message and returnsNone
. - Otherwise, it temporarily stores the current head node in
temp
. - Moves the
head
pointer to the second node (head.next
). - If the new head is not
None
, it updates itsprev
pointer toNone
to disconnect it from the old head. - Deletes the old head using
del temp
to free memory. - Returns the updated head node.
- If the list is empty (
- Purpose: Deletes the first node in the list and updates the
- print_list Function:
- Purpose: Traverses and prints the elements of the list.
- Starts from the
head
and iterates through the list using thenext
pointer. - Prints each node’s
data
, separated by spaces.
- Main Driver Code:
- Creates a hardcoded doubly linked list:
1 <-> 2 <-> 3
. - Calls
print_list
to display the original list. - Calls
del_head
to delete the first node. - Prints the updated list after the deletion.
- Creates a hardcoded doubly linked list:
2. Deletion at the End in a Doubly Linked List
Deleting a node at the end requires moving to the last node and updating the pointer of the second-to-last node.
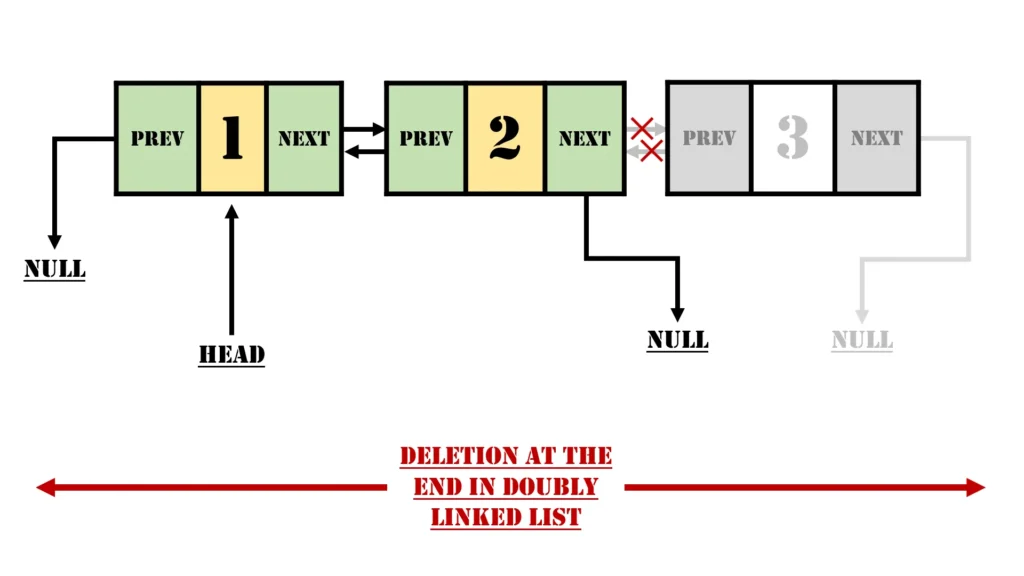
Steps:
- Check if the list is empty: If
head
isNULL
, there’s nothing to delete. - Traverse to the last node: Use a pointer, say
curr
, to iterate through the list untilcurr->next == NULL
. - Update the second-to-last node: Set the
next
pointer of the second-to-last node toNULL
usingcurr->prev->next = NULL
. - Free memory: Delete the last node (
curr
) to release allocated memory.
Example:
Input: DLL = 1 <-> 2 <-> 3
Output after deleting the last node: 1 <-> 2
Code Implementation In Python Programming Language
# Define the Node class for the Doubly Linked List
class Node:
def __init__(self, data):
self.data = data # Store the node's data
self.prev = None # Pointer to the previous node, initially None
self.next = None # Pointer to the next node, initially None
# Function to delete the last node of a Doubly Linked List
def del_last(head):
# Case 1: If the list is empty, return None
if head is None:
print("The list is empty, nothing to delete.")
return None
# Case 2: If the list contains only one node
if head.next is None:
print(f"Deleting Node with data {head.data} at the End.")
return None # After deletion, the list becomes empty
# Case 3: Traverse to the last node
curr = head
while curr.next is not None: # Move until the last node
curr = curr.next
# Update the previous node's next pointer to None
if curr.prev is not None:
print(f"Deleting Node with data {curr.data} at the End.")
curr.prev.next = None
# Return the head of the updated list
return head
# Function to print the Doubly Linked List
def print_list(head):
curr = head
print("Linked List:", end=" ")
while curr is not None: # Traverse through the list and print data
print(curr.data, end=" ")
curr = curr.next
print()
# Main function
if __name__ == "__main__":
# Create a hardcoded Doubly Linked List: 1 <-> 2 <-> 3
head = Node(1) # Create the first node with data 1
second = Node(2) # Create the second node with data 2
third = Node(3) # Create the third node with data 3
# Link the nodes together
head.next = second # Link the first node to the second
second.prev = head # Link the second node back to the first
second.next = third # Link the second node to the third
third.prev = second # Link the third node back to the second
# Print the original list
print("Original Linked List:", end=" ")
print_list(head)
# Delete the last node
head = del_last(head)
# Print the updated list after deletion
print("After Deleting the Last Node:", end=" ")
print_list(head)
Output
Original Linked List: 1 2 3
Deleting Node with data 3 at the End.
After Deleting the Last Node: 1 2
Time Complexity: O(n), where n is the number of nodes in the doubly linked list.
Auxiliary Space: O(1)
Step-by-Step Explanation
- Define the
Node
Class:- Each node in the Doubly Linked List contains
data
,prev
, andnext
attributes. data
: Stores the value of the node.prev
: Points to the previous node.next
: Points to the next node.
- Each node in the Doubly Linked List contains
- Function:
del_last(head)
:- Case 1: Checks if the list is empty (
head is None
). If true, it prints a message and returnsNone
. - Case 2: Handles the case where the list contains only one node (
head.next is None
). After deleting the node, the list becomes empty, so the function returnsNone
. - Case 3: Traverses the list to find the last node (
curr.next is None
). The loop moves thecurr
pointer until the last node is reached. - Updates the
next
pointer of the second-to-last node (curr.prev.next
) toNone
to detach the last node. - Prints a message specifying the data of the node being deleted.
- Case 1: Checks if the list is empty (
- Function:
print_list(head)
:- Traverses the list starting from the
head
and prints thedata
of each node, ensuring that the entire list is displayed.
- Traverses the list starting from the
- Main Function:
- Creates a hardcoded list with three nodes (
1 <-> 2 <-> 3
) and links them appropriately using theirprev
andnext
pointers. - Prints the original list.
- Deletes the last node by calling
del_last(head)
. - Prints the updated list after the deletion.
- Creates a hardcoded list with three nodes (
3. Deletion After a Given Node in a Doubly Linked List
This method involves deleting the node immediately following a specified node.
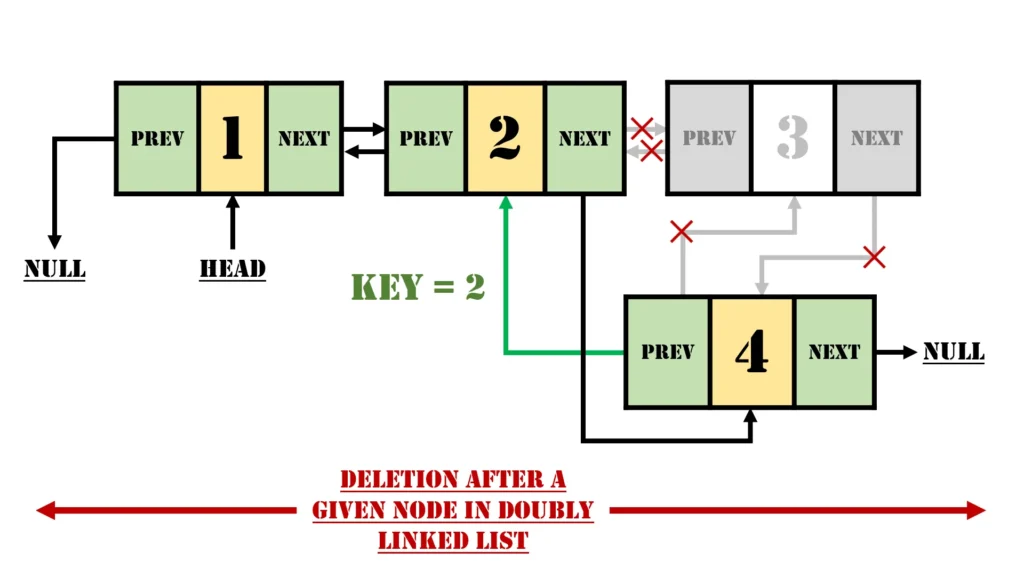
Steps:
- Locate the specified node: Use a pointer, say
curr
, to traverse the list untilcurr
points to the specified node. - Check if there is a next node: If
curr->next == NULL
, there’s no node to delete. Exit the function. - Set a pointer for the node to be deleted: Let
nodeDelete = curr->next
. - Update the next pointer of the current node: Set
curr->next = nodeDelete->next
. - Adjust the previous pointer of the next node: If
nodeDelete->next != NULL
, updatenodeDelete->next->prev = curr
. - Free memory: Delete
nodeDelete
to release allocated memory.
Example:
Input: DLL = 1 <-> 2 <-> 3 <-> 4
, Node = 2
Output after deleting the node after 2: 1 <-> 2 <-> 4
Code Implementation In Python Programming Language
class Node:
def __init__(self, data):
"""Initialize a Node with data, and set its next and prev pointers to None."""
self.data = data
self.next = None
self.prev = None
def delete_after(head, key):
"""
Deletes the node immediately following the node with the given key in the doubly linked list.
Parameters:
head (Node): The head of the doubly linked list.
key (int): The value of the node after which the deletion is to occur.
Returns:
Node: The updated head of the doubly linked list.
"""
# Initialize a pointer to traverse the list
curr = head
# Traverse the list to find the node with the specified key
while curr is not None:
if curr.data == key:
break
curr = curr.next
# Check if the key was found and if there is a node to delete after it
if curr is None or curr.next is None:
return head # Return the list unchanged if no deletion is possible
# Identify the node to be deleted
node_delete = curr.next
# Update the next pointer of the current node to skip the node to be deleted
curr.next = node_delete.next
# If the node to be deleted is not the last node, update the prev pointer of the next node
if node_delete.next is not None:
node_delete.next.prev = curr
return head # Return the updated list
def print_list(head):
"""
Prints the elements of the doubly linked list from head to tail.
Parameters:
head (Node): The head of the doubly linked list.
"""
curr = head
while curr is not None:
print(curr.data, end=" ")
curr = curr.next
print() # For a new line after printing the list
if __name__ == "__main__":
# Step 1: Create the doubly linked list
# 1 <-> 2 <-> 3 <-> 4
head = Node(1)
second = Node(2)
third = Node(3)
fourth = Node(4)
# Link the nodes together
head.next = second
second.prev = head
second.next = third
third.prev = second
third.next = fourth
fourth.prev = third
# Step 2: Print the original list
print("Original Linked List:")
print_list(head)
# Step 3: Perform deletion of the node after the node with data 2
print("\nDeleting Node with data 3 after Node with data 2:")
head = delete_after(head, 2)
# Step 4: Print the updated list
print_list(head)
Output:
Original Linked List:
1 2 3 4
Deleting Node with data 3 after Node with data 2:
1 2 4
Time Complexity: O(n), where n is the number of nodes in a doubly linked list.
Auxiliary Space: O(1)
4. Deletion Before a Given Node in a Doubly Linked List
In this case, we delete the node immediately preceding a specified node.
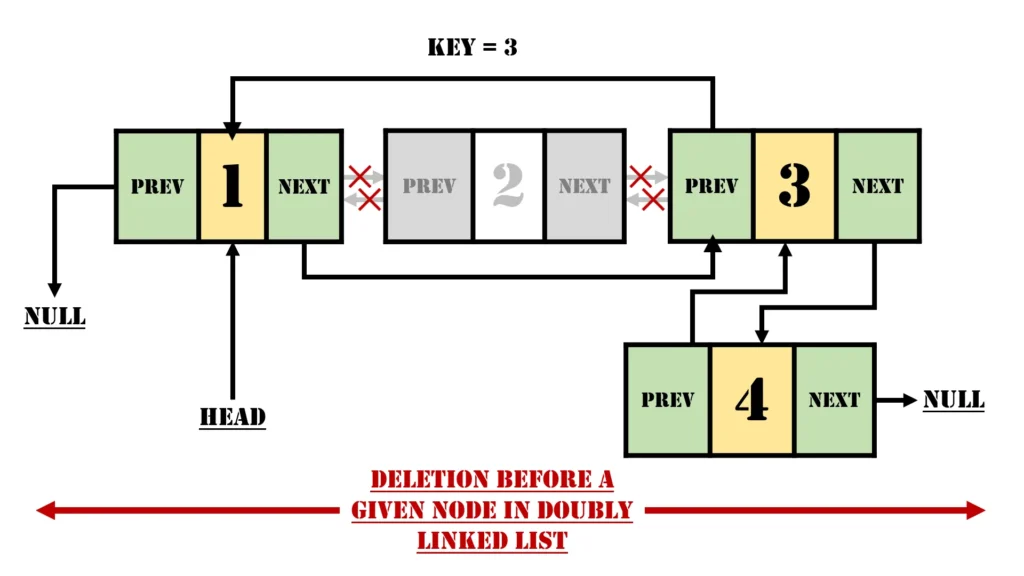
Steps:
- Locate the specified node: Use a pointer, say
curr
, to traverse the list untilcurr
points to the specified node. - Check if there is a previous node: If
curr->prev == NULL
, there’s no node to delete. Exit the function. - Set a pointer for the node to be deleted: Let
nodeDelete = curr->prev
. - Update the previous pointer of the current node: Set
curr->prev = nodeDelete->prev
. - Adjust the next pointer of the previous node: If
nodeDelete->prev != NULL
, updatenodeDelete->prev->next = curr
. - Free memory: Delete
nodeDelete
to release allocated memory.
Example:
Input: DLL = 1 <-> 2 <-> 3 <-> 4
, Node = 3
Output after deleting the node before 3: 1 <-> 3 <-> 4
Code Implementation In Python Programming Language
# Python Program to delete a node before a given node of a doubly linked list
class Node:
def __init__(self, data):
self.data = data
self.next = None
self.prev = None
def delete_before(head, key):
curr = head
# Traverse the list to find the node with the given key
while curr is not None:
if curr.data == key:
break
curr = curr.next
# If no such node exists or no node exists before the current node
if curr is None or curr.prev is None:
return head
# Node to be deleted
node_to_delete = curr.prev
# Update the prev pointer of the current node
curr.prev = node_to_delete.prev
# If node_to_delete is not the head, update its previous node's next pointer
if node_to_delete.prev is not None:
node_to_delete.prev.next = curr
else:
# If the node to delete is the head, update the head pointer
head = curr
return head
def print_list(head):
curr = head
while curr:
print(curr.data, end=" ")
curr = curr.next
print()
if __name__ == "__main__":
# Create a hardcoded doubly linked list: 1 <-> 2 <-> 3 <-> 4
head = Node(1)
second = Node(2)
third = Node(3)
fourth = Node(4)
# Link the nodes
head.next = second
second.prev = head
second.next = third
third.prev = second
third.next = fourth
fourth.prev = third
# Display the original list
print("Original Linked List: ", end="")
print_list(head)
# Delete the node before the node with data 3
head = delete_before(head, 3)
# Display the updated list
print("Deleting Node with data 2 before Node with data 3: ", end="")
print_list(head)
Output:
Original Linked List:
1 2 3 4
Deleting Node with data 2 before Node with data 3:
1 3 4
Time Complexity: O(n), where n is the number of nodes in a doubly linked list.
Auxiliary Space: O(1)
Explanation of the Code:
- Node Class:
- Each node contains
data
, anext
pointer, and aprev
pointer.
- Each node contains
- delete_before Function:
- Traverses the list to find the node with the key.
- Ensures there’s a node before the key node to delete.
- Updates the pointers of adjacent nodes to remove the target node.
- print_list Function:
- Traverses the list from
head
and prints the data of each node.
- Traverses the list from
- Main Section:
- Creates and links a doubly linked list.
- Prints the original list.
- Deletes the node before the specified key and prints the updated list.
5. Deletion at a Specific Position in a Doubly Linked List
To delete a node at a specific position, careful traversal and pointer adjustments are required.
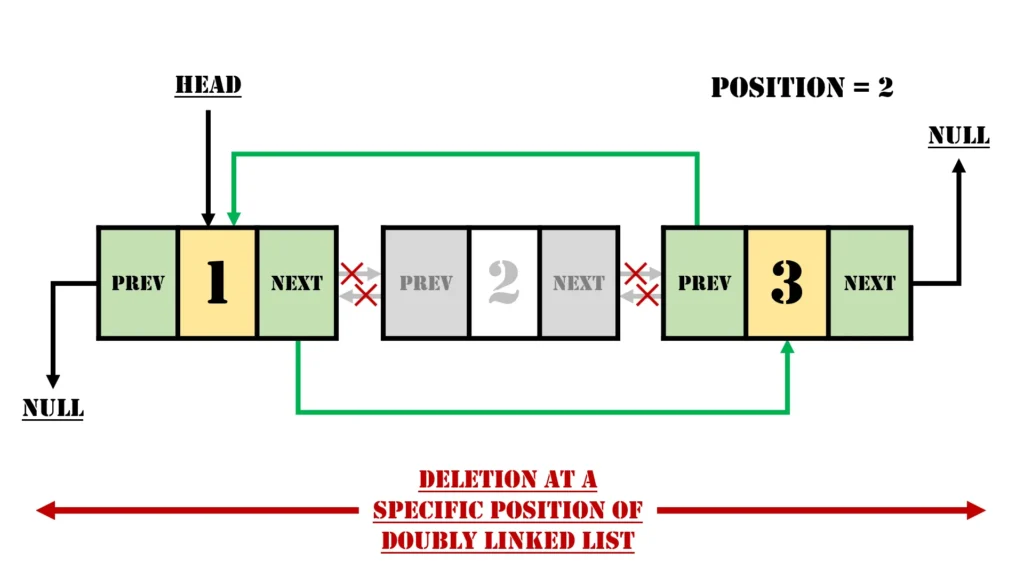
Steps:
- Traverse to the specified position: Use a pointer, say
curr
, to move to the node at the given position. - Check if the position is valid: If
curr == NULL
, the position is invalid. Exit the function. - Update pointers:
- If
curr
is not the head, setcurr->prev->next = curr->next
. - If
curr
is not the last node, setcurr->next->prev = curr->prev
.
- If
- Free memory: Delete
curr
to release allocated memory.
Example:
Input: DLL = 1 <-> 2 <-> 3
, Position = 2
Output after deleting the node at position 2: 1 <-> 3
Code Implementation In Python Programming Language
# Python program to delete a node at a specific position
# in a Doubly Linked List
class Node:
def __init__(self, data):
self.data = data
self.prev = None
self.next = None
# Function to delete a node at a specific position in the Doubly Linked List
def delete_at_position(head, position):
# If the list is empty, return the same head
if head is None:
return head
# Pointer to traverse the list
current = head
# Traverse to the node at the given position
for _ in range(1, position):
if current is None:
return head # Position is out of range
current = current.next
# If position is out of range
if current is None:
return head
# Update the previous node's next pointer
if current.prev is not None:
current.prev.next = current.next
# Update the next node's previous pointer
if current.next is not None:
current.next.prev = current.prev
# If the node to be deleted is the head
if head == current:
head = current.next
# Free the memory (handled automatically in Python)
current = None
# Return the updated head of the list
return head
# Function to print the doubly linked list
def print_list(head):
current = head
while current is not None:
print(current.data, end=" ")
current = current.next
print()
if __name__ == "__main__":
# Create a hardcoded doubly linked list: 1 <-> 2 <-> 3
head = Node(1)
second = Node(2)
third = Node(3)
# Linking the nodes
head.next = second
second.prev = head
second.next = third
third.prev = second
# Print the original list
print("Original Linked List:", end=" ")
print_list(head)
# Delete the node at position 2
head = delete_at_position(head, 2)
# Print the updated list
print("Deleting Node with data 2 at position 2:", end=" ")
print_list(head)
Output:
Original Linked List:
1 2 3
Deleting Node with data 2 at position 2:
1 3
Time Complexity: O(n), where n is the number of nodes in the doubly linked list.
Auxiliary Space: O(1)
Key Changes:
- Improved function and variable naming for better readability (
del_pos
→delete_at_position
,pos
→position
). - Added a comment about Python’s automatic memory management.
- Simplified traversal logic and ensured clarity in pointer updates.
Key Considerations When Deleting Nodes in a Doubly Linked List
- Memory Management: Always free the memory of the deleted node to prevent memory leaks.
- Boundary Cases: Handle cases where the list is empty, the position is invalid, or the node is at the beginning or end.
- Pointer Adjustments: Ensure all relevant pointers (
prev
andnext
) are correctly updated to maintain the list’s structure.
Advantages of Doubly Linked Lists in Deletion
A Doubly Linked List (DLL) provides unique advantages for deletion operations compared to other linear data structures, such as singly linked lists or arrays. The presence of both prev
and next
pointers in each node significantly enhances the efficiency and flexibility of node deletion.
Here are the key advantages of Doubly Linked Lists in deletion operations:
- Efficient Deletion at Any Position
- In a Doubly Linked List, nodes can be deleted efficiently from the beginning, middle, or end without needing to traverse the entire list:
- At the Beginning: Adjusting the
head
pointer and theprev
pointer of the new head is sufficient, making it an O(1) operation. - At the End: The
prev
pointer of the last node allows direct access to the second-to-last node, eliminating the need for full traversal. - In the Middle: Both the
next
pointer of the preceding node and theprev
pointer of the following node can be adjusted in constant time.
- At the Beginning: Adjusting the
- In a Doubly Linked List, nodes can be deleted efficiently from the beginning, middle, or end without needing to traverse the entire list:
- Bi-Directional Traversal
- The ability to traverse the list in both directions (forward and backward) makes locating nodes for deletion more flexible:
- If the target node is closer to the head, a forward traversal is efficient.
- If it’s closer to the tail, a backward traversal saves time.
- This bi-directional capability is particularly useful when deleting nodes near the end of a large list.
- The ability to traverse the list in both directions (forward and backward) makes locating nodes for deletion more flexible:
- No Need for Previous Node Tracking
- Unlike in a singly linked list, where deleting a node requires keeping track of the previous node during traversal, a Doubly Linked List inherently maintains this information via the
prev
pointer. This simplifies the deletion logic and reduces programming complexity. - For example:
- To delete a node, you can directly access its
prev
andnext
pointers without requiring additional traversal or auxiliary variables.
- To delete a node, you can directly access its
- Unlike in a singly linked list, where deleting a node requires keeping track of the previous node during traversal, a Doubly Linked List inherently maintains this information via the
- Simplified Deletion of Tail Nodes
- In a singly linked list, deleting the last node requires a full traversal to update the second-to-last node’s pointer. In a Doubly Linked List, the
prev
pointer of the last node provides immediate access to the second-to-last node, making tail deletion faster and more efficient.
- In a singly linked list, deleting the last node requires a full traversal to update the second-to-last node’s pointer. In a Doubly Linked List, the
- Robust Handling of Edge Cases
- The
prev
pointer in a Doubly Linked List helps to handle edge cases more effectively:- Empty List: You can easily check if the
head
isNULL
before performing deletion. - Single Node: Both the
prev
andnext
pointers of a single node will beNULL
, simplifying deletion logic. - Adjacent Nodes: Both forward and backward adjustments are straightforward, ensuring structural integrity.
- Empty List: You can easily check if the
- The
- Flexibility in Real-Time Applications
- Due to the ease of deletion, Doubly Linked Lists are preferred in applications requiring frequent modifications, such as:
- Dynamic Memory Management: Efficiently allocate and deallocate nodes.
- Undo/Redo Operations: Deleting and restoring recent changes is easier due to bi-directional linking.
- Cache Management: Removing nodes from the middle or tail is faster compared to arrays or singly linked lists.
- Due to the ease of deletion, Doubly Linked Lists are preferred in applications requiring frequent modifications, such as:
- Memory Utilization Trade-off
- While Doubly Linked Lists consume extra memory for the
prev
pointer, this trade-off is often justified by the significant performance gains in deletion operations. The added flexibility and efficiency outweigh the additional memory overhead in many practical scenarios.
- While Doubly Linked Lists consume extra memory for the
In conclusion, the Doubly Linked List provides a powerful and efficient structure for deletion operations. Its bi-directional linking, direct access to neighboring nodes, and reduced traversal requirements make it a versatile choice in dynamic applications where frequent node removal is necessary.
Related Articles
- Deletion in a Doubly Linked List with Implementation: A Comprehensive Guide
- Deletion at the Beginning in a Doubly Linked List: A Detailed Exploration
- Deletion after a given node in Doubly Linked List: A Comprehensive Guide
- Deleting a Node Before a Given Node in a Doubly Linked List: A Detailed Exploration
- Deletion at a Specific Position in a Doubly Linked List: A Detailed Exploration
- Deletion at the End in Doubly Linked List: A Comprehensive Exploration
Read More Articles
- Data Structure (DS) Array:
- Why the Analysis of Algorithms is Important?
- Worst, Average, and Best Case Analysis of Algorithms: A Comprehensive Guide
- Understanding Pointers in C Programming: A Comprehensive Guide
- Understanding Arrays in Data Structures: A Comprehensive Exploration
- Memory Allocation of an Array: An In-Depth Comprehensive Exploration
- Understanding Basic Operations in Arrays: A Comprehensive Guide
- Understanding 2D Arrays in Programming: A Comprehensive Guide
- Mapping a 2D Array to a 1D Array: A Comprehensive Exploration
- Data Structure Linked List:
- Understanding Linked Lists in Data Structures: A Comprehensive Exploration
- Types of Linked List: Detailed Exploration, Representations, and Implementations
- Understanding Singly Linked Lists: A Detailed Exploration
- Understanding Doubly Linked List: A Comprehensive Guide
- Operations of Doubly Linked List with Implementation: A Detailed Exploration
- Insertion in Doubly Linked List with Implementation: A Detailed Exploration
- Inserting a Node at the Beginning of a Doubly Linked List: A Detailed Exploration
- Inserting a Node After a Given Node in a Doubly Linked List: A Detailed Exploration
- Inserting a Node Before a Given Node in a Doubly Linked List: A Detailed Exploration
- Inserting a Node at a Specific Position in a Doubly Linked List: A Detailed Exploration
- Inserting a New Node at the End of a Doubly Linked List: A Detailed Exploration
- Deletion in a Doubly Linked List with Implementation: A Comprehensive Guide
- Deletion at the Beginning in a Doubly Linked List: A Detailed Exploration
- Deletion after a given node in Doubly Linked List: A Comprehensive Guide
- Deleting a Node Before a Given Node in a Doubly Linked List: A Detailed Exploration
- Deletion at a Specific Position in a Doubly Linked List: A Detailed Exploration
- Deletion at the End in Doubly Linked List: A Comprehensive Exploration
- Introduction to Circular Linked Lists: A Comprehensive Guide
- Understanding Circular Singly Linked Lists: A Comprehensive Guide
- Circular Doubly Linked List: A Comprehensive Guide
- Insertion in Circular Singly Linked List: A Comprehensive Guide
- Insertion in an Empty Circular Linked List: A Detailed Exploration
- Insertion at the Beginning in Circular Linked List: A Detailed Exploration
- Insertion at the End of a Circular Linked List: A Comprehensive Guide
- Insertion at a Specific Position in a Circular Linked List: A Detailed Exploration
- Deletion from a Circular Linked List: A Comprehensive Guide
- Deletion from the Beginning of a Circular Linked List: A Detailed Exploration
- Deletion at Specific Position in Circular Linked List: A Detailed Exploration
- Deletion at the End of a Circular Linked List: A Comprehensive Guide
- Searching in a Circular Linked List: A Comprehensive Exploration
Frequently Asked Questions (FAQs) About Deletion in Doubly Linked Lists
What is a Doubly Linked List, and how does it differ from a Singly Linked List?
A Doubly Linked List (DLL) is a type of linked list in which each node contains three components:
- Data: Stores the value of the node.
- Next Pointer (
next
): Points to the next node in the sequence. - Previous Pointer (
prev
): Points to the previous node in the sequence.
Key Differences:
- Singly Linked List (SLL): Nodes have only a
next
pointer, allowing traversal in a single direction. - Doubly Linked List: Nodes have both
next
andprev
pointers, enabling traversal in both forward and backward directions.
This bi-directional nature of DLL makes it more efficient for operations like deletion, especially for nodes located at the tail or middle of the list.
How is deletion in a Doubly Linked List more efficient than in a Singly Linked List?
Deletion in a DLL is more efficient because of its bi-directional linking:
- The
prev
pointer allows direct access to the node’s predecessor without needing a full traversal. - For deletion at the tail, the
prev
pointer of the last node provides immediate access to the second-to-last node. - For middle deletions, both
prev
andnext
pointers simplify pointer adjustments.
In contrast, Singly Linked Lists require a complete traversal to find the previous node, increasing the time complexity for certain deletions.
What are the steps to delete a node at the beginning of a Doubly Linked List?
Deleting the first node of a Doubly Linked List involves the following steps:
- Check if the list is empty: If
head == NULL
, there’s no node to delete. - Store the current head: Save the current
head
node in a temporary pointer, saytemp
. - Update the head pointer: Set
head
to the next node usinghead = head->next
. - Adjust the new head: If
head != NULL
, sethead->prev = NULL
. - Free memory: Delete the
temp
node to release its memory.
This operation ensures that the integrity of the list is maintained while efficiently removing the first node.
How do you delete a node at the end of a Doubly Linked List?
To delete the last node in a DLL:
- Check if the list is empty: If
head == NULL
, there’s nothing to delete. - Traverse to the last node: Use a pointer
curr
to find the node wherecurr->next == NULL
. - Update the second-to-last node: Set
curr->prev->next = NULL
to remove the last node from the list. - Free memory: Delete the
curr
node to release its allocated memory.
This process benefits from the prev
pointer, as it eliminates the need for a full backward traversal, unlike in Singly Linked Lists.
What is the process for deleting a node after a specific node in a Doubly Linked List?
To delete a node after a given node:
- Locate the specified node: Use a pointer, say
curr
, to find the node after which the deletion should occur. - Check if there is a next node: If
curr->next == NULL
, there’s no node to delete. - Set a pointer to the node to be deleted: Let
nodeDelete = curr->next
. - Update the pointers:
- Set
curr->next = nodeDelete->next
. - If
nodeDelete->next != NULL
, updatenodeDelete->next->prev = curr
.
- Set
- Free memory: Delete the
nodeDelete
node.
This approach maintains the integrity of the list by correctly updating the surrounding nodes’ pointers.
How can you delete a node before a specific node in a Doubly Linked List?
To delete a node before a given node:
- Locate the specified node: Use a pointer
curr
to point to the target node. - Check if there is a previous node: If
curr->prev == NULL
, the node is the head, and there’s no node to delete before it. - Set a pointer to the node to be deleted: Let
nodeDelete = curr->prev
. - Update pointers:
- Set
curr->prev = nodeDelete->prev
. - If
nodeDelete->prev != NULL
, updatenodeDelete->prev->next = curr
.
- Set
- Free memory: Delete
nodeDelete
to release memory.
This operation is efficient because the prev
pointer provides direct access to the preceding node.
What are the steps for deleting a node at a specific position in a Doubly Linked List?
To delete a node at a given position:
- Traverse to the specified position: Use a pointer, say
curr
, to move to the node at the given position. - Check if the position is valid: If
curr == NULL
, the position is invalid. - Update pointers:
- If
curr->prev != NULL
, setcurr->prev->next = curr->next
. - If
curr->next != NULL
, setcurr->next->prev = curr->prev
.
- If
- Free memory: Delete the
curr
node.
This method efficiently handles both edge and middle cases due to the presence of prev
and next
pointers.
Why is the memory overhead of a Doubly Linked List justified?
Although DLL requires extra memory for the prev
pointer in each node, this overhead is justified because:
- It significantly improves the efficiency of deletion, insertion, and traversal operations.
- Deleting tail nodes or nodes at arbitrary positions is faster than in Singly Linked Lists, where a full traversal might be required.
- The bi-directional nature of DLL allows flexibility for dynamic applications like undo/redo systems or cache management.
In many cases, the performance benefits outweigh the additional memory usage.
What are the advantages of Doubly Linked Lists in real-world applications?
Doubly Linked Lists are widely used in various real-world scenarios due to their flexibility:
- Dynamic Memory Management: Efficient allocation and deallocation of nodes.
- Undo/Redo Functionality: The bi-directional traversal simplifies the implementation of undo/redo operations in text editors or version control systems.
- Cache Management: DLL is used in algorithms like Least Recently Used (LRU) Cache, where fast deletion and insertion are critical.
- Navigation Systems: Forward and backward traversal in lists, such as in browser histories or media playlists.
These advantages make DLL an indispensable data structure for dynamic and complex applications.
What are the key considerations when deleting nodes in a Doubly Linked List?
When performing deletion operations in a Doubly Linked List, keep the following in mind:
- Handle Empty Lists: Always check if the
head == NULL
before attempting deletion. - Free Memory: Ensure that the memory allocated for the deleted node is properly freed to prevent memory leaks.
- Adjust Surrounding Pointers: Carefully update the
prev
andnext
pointers of neighboring nodes to maintain the list structure. - Edge Cases: Handle boundary scenarios, such as deleting the head, tail, or the only node in the list.
- Validity Checks: Ensure that the specified node or position exists to avoid segmentation faults or undefined behavior.