In the realm of Data Structures, the Circular Linked List (CLL) is a unique variation of the Linked List, characterized by its circular nature. Unlike the conventional Singly Linked List, the last node in a Circular Linked List points back to the head, creating a continuous loop. This structure makes traversal and specific operations different and often more efficient in certain scenarios. One such operation is searching for a specific value within the CLL. This guide delves into the detailed process, the underlying logic, and the implementation of searching in a Circular Linked List.
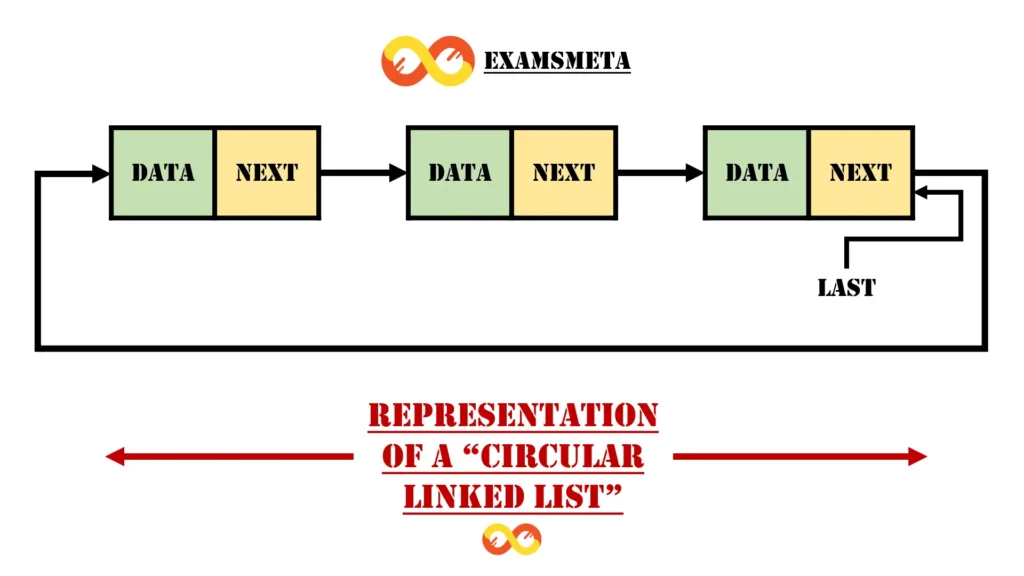
Table of Contents
Understanding the Searching Process in Circular Linked Lists
Searching in a Circular Linked List follows a methodology akin to searching in a regular Linked List. However, due to the circular structure, special care must be taken to avoid infinite loops during traversal. The steps involved in searching can be outlined as follows:
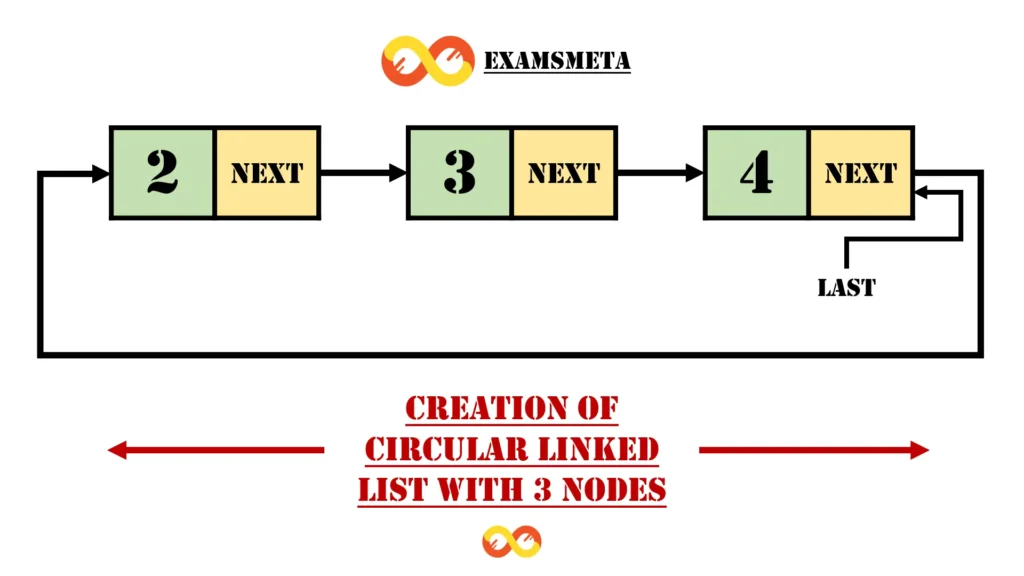
Step 1: Handle the Base Case: An Empty List
Before proceeding, the first task is to check if the list is empty. In programming terms, this is done by verifying whether the head
node of the CLL is null
(or None
in Python). If the list is empty, it is impossible to find any element, and the search operation immediately returns false
.
Step 2: Initiate Traversal from the Head Node
Once it is established that the list contains nodes, traversal begins at the head
node, which can be accessed as the last->next
node. A pointer, often named curr
, is initialized to this starting node. This pointer will be used to iterate through the list.
Step 3: Traverse the List While Tracking the Starting Point
The traversal continues by moving the pointer from one node to the next using the node’s next
reference. To avoid looping infinitely in the circular structure, we must carefully track when we return to the starting node (head
).
Step 4: Compare Each Node’s Data with the Target Value
During each iteration, the data stored in the current node is compared with the target value (denoted as K
). If a match is found, the function returns true
, indicating that the value exists in the list.
Step 5: Check the Last Node Explicitly
Before concluding the traversal, it is crucial to check the last node. Since the traversal halts upon returning to the head
, failing to explicitly check the last node could result in missing the target value if it resides there.
Step 6: Conclude the Search
If the pointer completes a full circle and reaches the head
again without finding the target value, the function returns false
, signifying that the value is not present in the list.
Detailed Example and Visualization
Let us consider the Circular Linked List:CList = 6 -> 5 -> 4 -> 3 -> 2
, and the target value K = 3.
Case 1: Element Found
- Start traversal at
head
(node with value6
). - Compare
6
with3
– no match, move to the next node. - Compare
5
with3
– no match, move to the next node. - Compare
4
with3
– no match, move to the next node. - Compare
3
with3
– match found. Returntrue
.
Output: Found
Case 2: Element Not Found
Now consider K = 1.
- Start traversal at
head
(node with value6
). - Compare
6
with1
– no match, move to the next node. - Compare
5
with1
– no match, move to the next node. - Compare
4
with1
– no match, move to the next node. - Compare
3
with1
– no match, move to the next node. - Compare
2
with1
– no match, reach back tohead
.
Output: Not Found
Algorithm for Searching in a Circular Linked List
The logic outlined above can be translated into a generic algorithm:
- Check if the list is empty:
- If
head == null
, returnfalse
.
- If
- Initialize pointers:
- Set
curr = head
.
- Set
- Traverse the list:
- While
curr != head
or first iteration:- Compare
curr.data
with the target valueK
. - If match found, return
true
. - Move to the next node using
curr = curr.next
.
- Compare
- While
- Final check on the last node:
- Compare the data in
last
withK
.
- Compare the data in
- Return
false
if no match is found.
Python Programming Implementation
Below is an example of the algorithm implemented in Python:
class Node:
def __init__(self, data):
self.data = data
self.next = None
class CircularLinkedList:
def __init__(self):
self.head = None
def append(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
self.head.next = self.head
else:
temp = self.head
while temp.next != self.head:
temp = temp.next
temp.next = new_node
new_node.next = self.head
def search(self, key):
if not self.head:
return False
curr = self.head
while True:
if curr.data == key:
return True
curr = curr.next
if curr == self.head:
break
return False
# Example Usage
cll = CircularLinkedList()
for val in [6, 5, 4, 3, 2]:
cll.append(val)
print("3 Found" if cll.search(3) else "3 Not Found")
print("1 Found" if cll.search(1) else "1 Not Found")
Output
3 Found
1 Not Found
Code Implementation In Multiple Programming Languages
Here is the detailed implementation of the code of searching in a circular linked list while maintaining its structure and efficiency in multiple programming languages. {Like: C, C++, C#, Java, JavaScript, and Python}
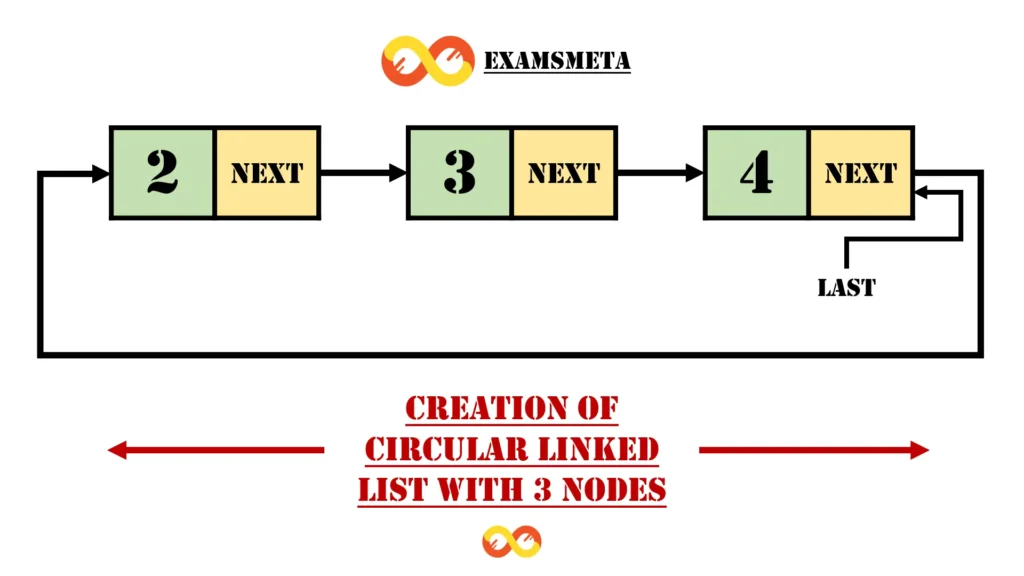
C Programming
#include <stdio.h>
#include <stdlib.h>
// Definition of the Node structure
struct Node {
int data;
struct Node *next;
};
// Function to search for a specific value in the circular linked list
int search(struct Node *last, int key) {
if (last == NULL) {
// If the list is empty
return 0;
}
struct Node *head = last->next;
struct Node *curr = head;
// Traverse the list to find the key
do {
if (curr->data == key) {
// Key found
return 1;
}
curr = curr->next;
} while (curr != head);
// Key not found
return 0;
}
// Function to print the circular linked list
void printList(struct Node *last) {
if (last == NULL) return;
struct Node *head = last->next;
struct Node *curr = head;
do {
printf("%d ", curr->data);
curr = curr->next;
} while (curr != head);
printf("\n");
}
// Function to create a new node
struct Node *createNode(int value) {
struct Node *temp = (struct Node *)malloc(sizeof(struct Node));
temp->data = value;
temp->next = NULL;
return temp;
}
int main() {
// Create circular linked list: 2, 3, 4
struct Node *first = createNode(2);
struct Node *second = createNode(3);
struct Node *third = createNode(4);
first->next = second;
second->next = third;
third->next = first;
struct Node *last = third;
printf("Original list: ");
printList(last);
// Search for a specific value
int key = 3;
if (search(last, key)) {
printf("Value %d found in the list.\n", key);
} else {
printf("Value %d not found in the list.\n", key);
}
return 0;
}
C++ Implementation
#include <iostream>
using namespace std;
// Definition of the Node structure
struct Node {
int data;
Node *next;
};
// Function to search for a specific value in the circular linked list
bool search(Node *last, int key) {
if (last == nullptr) {
// If the list is empty
return false;
}
Node *head = last->next;
Node *curr = head;
// Traverse the list to find the key
do {
if (curr->data == key) {
// Key found
return true;
}
curr = curr->next;
} while (curr != head);
// Key not found
return false;
}
// Function to print the circular linked list
void printList(Node *last) {
if (last == nullptr) return;
Node *head = last->next;
Node *curr = head;
do {
cout << curr->data << " ";
curr = curr->next;
} while (curr != head);
cout << endl;
}
// Function to create a new node
Node *createNode(int value) {
Node *temp = new Node();
temp->data = value;
temp->next = nullptr;
return temp;
}
int main() {
// Create circular linked list: 2, 3, 4
Node *first = createNode(2);
Node *second = createNode(3);
Node *third = createNode(4);
first->next = second;
second->next = third;
third->next = first;
Node *last = third;
cout << "Original list: ";
printList(last);
// Search for a specific value
int key = 3;
if (search(last, key)) {
cout << "Value " << key << " found in the list." << endl;
} else {
cout << "Value " << key << " not found in the list." << endl;
}
return 0;
}
C# Implementation
using System;
class Node {
public int Data;
public Node Next;
}
class Program {
static bool Search(Node last, int key) {
if (last == null) return false;
Node head = last.Next;
Node curr = head;
do {
if (curr.Data == key) {
return true;
}
curr = curr.Next;
} while (curr != head);
return false;
}
static void PrintList(Node last) {
if (last == null) return;
Node head = last.Next;
Node curr = head;
do {
Console.Write(curr.Data + " ");
curr = curr.Next;
} while (curr != head);
Console.WriteLine();
}
static Node CreateNode(int value) {
return new Node { Data = value, Next = null };
}
static void Main() {
Node first = CreateNode(2);
Node second = CreateNode(3);
Node third = CreateNode(4);
first.Next = second;
second.Next = third;
third.Next = first;
Node last = third;
Console.WriteLine("Original list:");
PrintList(last);
int key = 3;
if (Search(last, key)) {
Console.WriteLine($"Value {key} found in the list.");
} else {
Console.WriteLine($"Value {key} not found in the list.");
}
}
}
Java Implementation
class Node {
int data;
Node next;
Node(int data) {
this.data = data;
this.next = null;
}
}
public class CircularLinkedList {
// Function to search for a specific value
public static boolean search(Node last, int key) {
if (last == null) {
// If the list is empty
return false;
}
Node head = last.next;
Node curr = head;
// Traverse the list to find the key
do {
if (curr.data == key) {
return true; // Key found
}
curr = curr.next;
} while (curr != head);
return false; // Key not found
}
// Function to print the circular linked list
public static void printList(Node last) {
if (last == null) return;
Node head = last.next;
Node curr = head;
do {
System.out.print(curr.data + " ");
curr = curr.next;
} while (curr != head);
System.out.println();
}
public static void main(String[] args) {
// Create circular linked list: 2, 3, 4
Node first = new Node(2);
Node second = new Node(3);
Node third = new Node(4);
first.next = second;
second.next = third;
third.next = first;
Node last = third;
System.out.println("Original list:");
printList(last);
// Search for a specific value
int key = 3;
if (search(last, key)) {
System.out.println("Value " + key + " found in the list.");
} else {
System.out.println("Value " + key + " not found in the list.");
}
}
}
JavaScript Implementation
class Node {
constructor(data) {
this.data = data;
this.next = null;
}
}
function search(last, key) {
if (!last) {
// If the list is empty
return false;
}
let head = last.next;
let curr = head;
// Traverse the list to find the key
do {
if (curr.data === key) {
return true; // Key found
}
curr = curr.next;
} while (curr !== head);
return false; // Key not found
}
function printList(last) {
if (!last) return;
let head = last.next;
let curr = head;
do {
process.stdout.write(curr.data + " ");
curr = curr.next;
} while (curr !== head);
console.log();
}
// Main Execution
const first = new Node(2);
const second = new Node(3);
const third = new Node(4);
first.next = second;
second.next = third;
third.next = first;
const last = third;
console.log("Original list:");
printList(last);
const key = 3;
if (search(last, key)) {
console.log(`Value ${key} found in the list.`);
} else {
console.log(`Value ${key} not found in the list.`);
}
Python Implementation
class Node:
def __init__(self, data):
self.data = data
self.next = None
def search(last, key):
if not last:
# If the list is empty
return False
head = last.next
curr = head
# Traverse the list to find the key
while True:
if curr.data == key:
return True # Key found
curr = curr.next
if curr == head:
break
return False # Key not found
def print_list(last):
if not last:
return
head = last.next
curr = head
while True:
print(curr.data, end=" ")
curr = curr.next
if curr == head:
break
print()
# Main Execution
first = Node(2)
second = Node(3)
third = Node(4)
first.next = second
second.next = third
third.next = first
last = third
print("Original list:")
print_list(last)
key = 3
if search(last, key):
print(f"Value {key} found in the list.")
else:
print(f"Value {key} not found in the list.")
Output
The output of the code in all the programming languages will be as follows, assuming the circular linked list contains the values 2 -> 3 -> 4
and you are searching for the key 3
:
Original list: 2 3 4
Value 3 found in the list.
If the key is changed to 5
(a value not in the list), the output would be:
Original list: 2 3 4
Value 5 not found in the list.
Detailed Explanation for All Implementations
- Node Class/Struct Definition:
- Each node stores a
data
value and anext
pointer/reference to the next node. - In Python and JavaScript, constructors initialize the node.
- In languages like C/C++, structs define the data members and pointers.
- Each node stores a
- Circular Nature:
- The last node’s
next
pointer points back to the first node, creating a circular structure. - This enables seamless traversal without needing
null
checks.
- The last node’s
- Search Function:
- Start with the head node (
last.next
). - Use a loop (
do-while
in C/C++, JavaScript, and Java;while True
in Python) to traverse the list. - Compare the
data
of each node with the target value (key
). - Terminate traversal when you return to the head.
- Start with the head node (
- Print Function:
- Iterates through the circular list, starting from the head.
- Prints the
data
of each node until the pointer returns to the head.
- Main Execution:
- Creates a circular linked list manually by linking nodes and making the last node point to the first.
- Calls the
print
function to display the list. - Searches for a specific key and prints whether it was found or not.
These implementations ensure that the functionality is consistent across languages.
Informative Table: Detailed Overview of Searching in a Circular Linked List
Aspect | Description |
---|---|
Definition | A Circular Linked List (CLL) is a data structure where the last node points back to the head node, creating a circular structure. It can be singly linked (each node has one pointer to the next node) or doubly linked (each node has two pointers: next and prev ). |
Key Characteristics | – No null pointer exists at the end. – The list forms a continuous loop. – Traversal starts at the head and ends when the pointer returns to the head. |
Use Case for Searching | Searching in a CLL involves locating a specific value (K ) in the list. It requires handling the circular nature to avoid infinite loops. |
Steps of the Algorithm | 1. Check if the list is empty: If head == null , return false . 2. Initialize traversal: Start with a pointer (curr ) set to the head. 3. Traverse and compare: Check each node’s data with the target value (K ). 4. Terminate loop: Stop when pointer returns to head . |
Base Case | If the Circular Linked List is empty, indicated by a null or None head , the function immediately returns false . |
Handling the Circular Nature | – Maintain a starting reference to the head node. – During traversal, check if the pointer returns to the head to avoid infinite loops. |
Traversal | Move the pointer from one node to the next using the next reference. Repeat until the pointer either finds the target value or returns to the starting node (head ). |
Key Checks | – Compare the target value (K ) with the data of the current node. – Explicitly check the last node before completing traversal. |
Output Scenarios | – Found: If a node with the target value (K ) is located, the algorithm returns true . – Not Found: If traversal completes a full loop without finding the value, return false . |
Time Complexity | – Worst-case scenario: O(n), where n is the number of nodes in the list. – Linear traversal is required as each node must be checked. |
Space Complexity | O(1), as no additional space is required beyond the traversal pointer. |
Advantages of CLL in Searching | – Seamless traversal due to the circular nature. – No explicit check for null nodes, simplifying traversal logic. |
Drawbacks of CLL in Searching | – Risk of infinite loops if the circular structure is not handled properly. – Linear search requires O(n) time in the worst case. |
Algorithm in Pseudocode | <br> If head == null: <br> return False <br> curr = head <br> While True: <br> If curr.data == K: <br> return True <br> curr = curr.next <br> If curr == head: <br> break <br> return False |
Example | Input: CList = 6->5->4->3->2, K = 3 Output: Found Explanation: Traversal checks 6, 5, 4, and finds the match at node 3. |
Programming Languages | – Python: Classes and pointers. – C/C++: Structs and pointers. – Java: Objects and references. – JavaScript: Objects with next references. |
Real-World Applications | – Round Robin Scheduling: In operating systems, tasks are managed cyclically. – Multimedia Playlists: Tracks loop continuously. – Networking Protocols: Circular structures are used to manage resources in token-passing systems. |
Additional Notes | – Ensure robust testing to avoid infinite loops. – Handle edge cases such as empty lists or lists with only one node. |
This table provides a structured summary of searching in a Circular Linked List, covering all essential details.
Conclusion
Circular Linked Lists are efficient data structures with unique properties that require thoughtful handling of operations like searching. By carefully tracking traversal and utilizing the circular nature of the list, we can efficiently search for any value. Understanding and implementing such operations solidifies foundational knowledge of data structures, a cornerstone of computer science and programming.
Related Articles
- Introduction to Circular Linked Lists: A Comprehensive Guide
- Understanding Circular Singly Linked Lists: A Comprehensive Guide
- Circular Doubly Linked List: A Comprehensive Guide
- Insertion in Circular Singly Linked List: A Comprehensive Guide
- Insertion in an Empty Circular Linked List: A Detailed Exploration
- Insertion at the Beginning in Circular Linked List: A Detailed Exploration
- Insertion at the End of a Circular Linked List: A Comprehensive Guide
- Insertion at a Specific Position in a Circular Linked List: A Detailed Exploration
- Deletion from a Circular Linked List: A Comprehensive Guide
- Deletion from the Beginning of a Circular Linked List: A Detailed Exploration
- Deletion at Specific Position in Circular Linked List: A Detailed Exploration
- Deletion at the End of a Circular Linked List: A Comprehensive Guide
- Searching in a Circular Linked List: A Comprehensive Exploration
Read More Articles
- Data Structure (DS) Array:
- Why the Analysis of Algorithms is Important?
- Worst, Average, and Best Case Analysis of Algorithms: A Comprehensive Guide
- Understanding Pointers in C Programming: A Comprehensive Guide
- Understanding Arrays in Data Structures: A Comprehensive Exploration
- Memory Allocation of an Array: An In-Depth Comprehensive Exploration
- Understanding Basic Operations in Arrays: A Comprehensive Guide
- Understanding 2D Arrays in Programming: A Comprehensive Guide
- Mapping a 2D Array to a 1D Array: A Comprehensive Exploration
- Data Structure Linked List:
- Understanding Linked Lists in Data Structures: A Comprehensive Exploration
- Types of Linked List: Detailed Exploration, Representations, and Implementations
- Understanding Singly Linked Lists: A Detailed Exploration
- Understanding Doubly Linked List: A Comprehensive Guide
- Operations of Doubly Linked List with Implementation: A Detailed Exploration
- Insertion in Doubly Linked List with Implementation: A Detailed Exploration
- Inserting a Node at the beginning of a Doubly Linked List: A Detailed Exploration
- Inserting a Node After a Given Node in a Doubly Linked List: A Detailed Exploration
- Inserting a Node Before a Given Node in a Doubly Linked List: A Detailed Exploration
- Inserting a Node at a Specific Position in a Doubly Linked List: A Detailed Exploration
- Inserting a New Node at the End of a Doubly Linked List: A Detailed Exploration
- Deletion in a Doubly Linked List with Implementation: A Comprehensive Guide
- Deletion at the Beginning in a Doubly Linked List: A Detailed Exploration
- Deletion after a given node in Doubly Linked List: A Comprehensive Guide
- Deleting a Node Before a Given Node in a Doubly Linked List: A Detailed Exploration
- Deletion at a Specific Position in a Doubly Linked List: A Detailed Exploration
- Deletion at the End in Doubly Linked List: A Comprehensive Exploration
- Introduction to Circular Linked Lists: A Comprehensive Guide
- Understanding Circular Singly Linked Lists: A Comprehensive Guide
- Circular Doubly Linked List: A Comprehensive Guide
- Insertion in Circular Singly Linked List: A Comprehensive Guide
- Insertion in an Empty Circular Linked List: A Detailed Exploration
- Insertion at the Beginning in Circular Linked List: A Detailed Exploration
- Insertion at the End of a Circular Linked List: A Comprehensive Guide
- Insertion at a Specific Position in a Circular Linked List: A Detailed Exploration
- Deletion from a Circular Linked List: A Comprehensive Guide
- Deletion from the Beginning of a Circular Linked List: A Detailed Exploration
- Deletion at Specific Position in Circular Linked List: A Detailed Exploration
- Deletion at the End of a Circular Linked List: A Comprehensive Guide
- Searching in a Circular Linked List: A Comprehensive Exploration
Frequently Asked Questions (FAQs)
What is a Circular Linked List (CLL)?
A Circular Linked List (CLL) is a specialized version of a Linked List in which the last node does not point to null
(or None
in Python) but instead points back to the head node. This forms a circular structure, making traversal and certain operations more efficient in some scenarios.
For instance, in a Circular Singly Linked List, each node contains two elements:
- Data, which stores the value.
- Next, which points to the next node in the sequence.
The circular connection ensures there is no endpoint in the list, unlike a traditional Singly Linked List, where the last node points to null
.
Why is searching different in a Circular Linked List compared to a regular Linked List?
In a regular linked list, traversal ends when the pointer encounters null
, signaling the end of the list. However, in a Circular Linked List, there is no null
reference; the nodes are connected in a loop. Therefore:
- A starting point must be identified to ensure the list isn’t traversed indefinitely.
- Special care is required to track when traversal has completed a full circle.
- The algorithm must explicitly check the last node, as the loop ends upon reaching the starting node (head) again.
These considerations make searching in a Circular Linked List unique.
How does the algorithm for searching in a Circular Linked List work?
The algorithm involves the following steps:
- Check if the list is empty: If the
head
isnull
, immediately returnfalse
. - Start traversal from the head: Use a pointer (
curr
) initialized to thehead
node. - Traverse the list: Compare the value at each node with the target value (
K
). If a match is found, returntrue
. - Handle circular nature: Track when the traversal completes a full loop by checking if the pointer returns to the
head
. - Return false if no match is found after a full loop.
What happens if the Circular Linked List is empty?
If the Circular Linked List is empty, meaning there are no nodes, the head
pointer will be null
(or None
in Python). In such cases:
- The search function immediately returns
false
, as there are no elements to traverse or match. - No iteration or additional checks are performed.
This is the base case in the search algorithm.
How do you detect the end of traversal in a Circular Linked List?
Since a Circular Linked List loops back to the head
node, traversal ends when the pointer:
- Returns to the
head
node after one complete cycle. - This requires initializing a starting pointer (
curr
) at thehead
and comparing it during each iteration to ensure the loop does not continue indefinitely.
Using this approach ensures all nodes are checked exactly once during the search.
How do you check the last node in a Circular Linked List?
The last node in a Circular Linked List is directly linked to the head
. To ensure it is not missed:
- Compare the target value (
K
) with the data of the node just before the pointer returns to thehead
. - This step is implicitly covered in the main traversal loop but is particularly important if additional conditions are applied during traversal.
Can the search algorithm be implemented in all programming languages?
Yes, the search algorithm for Circular Linked Lists can be implemented in most programming languages, including:
- Python: Using classes and pointers for node representation.
- C/C++: Using structs and pointers.
- Java: Using objects and references.
- JavaScript: Using objects and
next
pointers.
The core logic remains the same, but the syntax and data structure implementation vary depending on the language.
Is searching in a Circular Linked List more efficient than in a regular Linked List?
Efficiency depends on the context:
- In a Circular Linked List, all nodes can be checked seamlessly in a loop without encountering
null
or additional conditions. - However, both types of lists require O(n) time complexity for searching in the worst case (traversing all nodes).
The circular structure provides advantages in other operations (e.g., insertion and deletion), but for searching, the efficiency is comparable.
How do you ensure infinite loops do not occur during traversal?
To prevent infinite loops:
- Always track the starting node (
head
) during traversal. - Use a condition to terminate the loop when the pointer (
curr
) returns to thehead
.
For example, in Python:
while True:
if curr == head:
break
Can the search algorithm handle duplicate values in the list?
Yes, the search algorithm can handle duplicates. However:
- The current implementation stops at the first match and returns
true
. - If all occurrences of the value need to be identified, the algorithm must be modified to continue traversal even after finding a match.
What happens if the target value is in the last node of the list?
If the target value resides in the last node, it will still be detected during traversal because the algorithm loops back to the head
only after checking the last node’s data. This is ensured by:
- Traversing until the pointer (
curr
) equals thehead
.
How can we modify the search algorithm to count occurrences of the target value?
To count all occurrences of the target value (K
):
- Introduce a counter variable (
count
) initialized to 0. - Increment
count
each time the value at the current node matchesK
. - Continue traversal until the pointer returns to the
head
.
For example:
def count_occurrences(self, key):
if not self.head:
return 0
curr = self.head
count = 0
while True:
if curr.data == key:
count += 1
curr = curr.next
if curr == self.head:
break
return count
Can the search algorithm be applied to Circular Doubly Linked Lists?
Yes, the algorithm is applicable to Circular Doubly Linked Lists (CDLL) with slight modifications:
- Traversal can move in both forward (
next
) and backward (prev
) directions. - The basic logic of detecting a match remains unchanged.
Are there specific use cases for Circular Linked Lists in real-world applications?
Yes, Circular Linked Lists are used in scenarios where:
- Continuous looping is required, such as in multimedia playlists.
- Processes need cyclic iteration, like in Round Robin Scheduling in operating systems.
- Memory-constrained systems where the absence of
null
pointers simplifies management.
Can the search algorithm be optimized for larger lists?
For larger lists, optimization techniques include:
- Using hashing or auxiliary data structures to store node data for faster lookup.
- Applying specific traversal techniques based on patterns in data distribution.
However, the inherent linear nature of traversal means the worst-case complexity remains O(n).