Introduction to Directed Graphs
A directed graph, or digraph, is a specialized form of a graph where each edge has a specific direction, indicating a one-way relationship between the two vertices it connects. Unlike undirected graphs, where edges represent bidirectional connections, a directed graph provides additional detail about how nodes relate to one another in a structured and often hierarchical manner. This structural complexity gives directed graphs a wide array of applications, from social networks to project management, where understanding the direction of relationships is crucial.
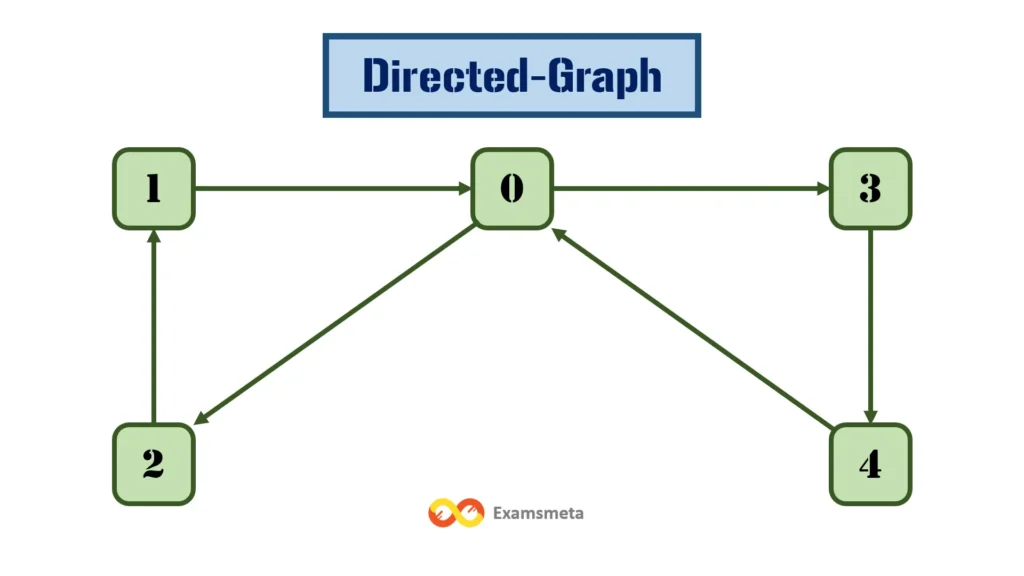
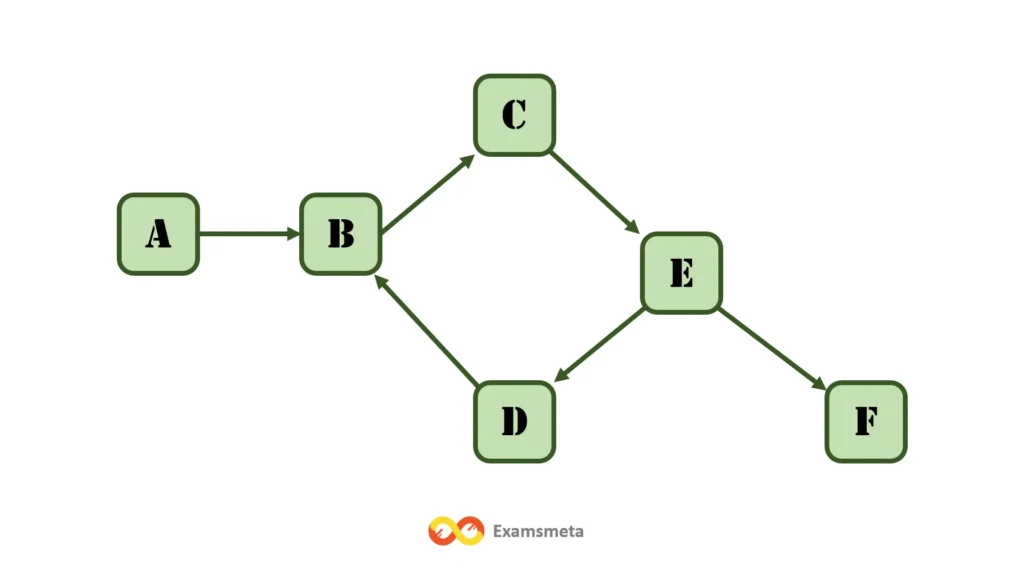
Table of Contents
Characteristics of Directed Graphs
Directed graphs possess several important characteristics that distinguish them from undirected graphs. These characteristics make them suitable for modeling many real-world problems where directionality is a key feature.
1. Directed Edges
The primary feature of a directed graph is that the edges have direction. Each edge points from one vertex (node) to another, forming a one-way relationship. For instance, if an edge connects vertex A to vertex B, the relationship implies that A leads to B, but not necessarily the other way around. The reverse relationship would need to be represented by another directed edge pointing from B to A.
In mathematical terms, a directed graph G is represented as G = (V, E), where:
- V is a set of vertices.
- E is a set of ordered pairs of vertices, representing directed edges.
For example, in a directed graph where A → B and B → C, we can traverse the graph from A to C, but we cannot move backward unless there are additional edges such as C → B or B → A.
2. Indegree and Outdegree
In a directed graph, each vertex has two specific measures of connectivity:
- Indegree: The number of incoming edges to a vertex. This indicates how many other vertices have connections pointing to this vertex.
- Outdegree: The number of outgoing edges from a vertex. This indicates how many vertices this vertex points to.
For instance, in a social network modeled as a directed graph, if person A follows person B, A has an outdegree of 1 (indicating they are following one person), and B has an indegree of 1 (indicating that one person is following them).
Mathematically, for a vertex v:
- Indegree(v): The number of edges directed towards v.
- Outdegree(v): The number of edges directed from v.
# Example in Python for calculating Indegree and Outdegree
class DirectedGraph:
def __init__(self):
self.graph = {}
def add_edge(self, u, v):
if u in self.graph:
self.graph[u].append(v)
else:
self.graph[u] = [v]
def indegree(self, v):
count = 0
for u in self.graph:
if v in self.graph[u]:
count += 1
return count
def outdegree(self, v):
return len(self.graph.get(v, []))
# Example usage:
g = DirectedGraph()
g.add_edge('A', 'B')
g.add_edge('B', 'C')
g.add_edge('A', 'C')
print(f"Indegree of C: {g.indegree('C')}")
print(f"Outdegree of A: {g.outdegree('A')}")
# Output...
# Indegree of C: 2
# Outdegree of A: 2
In the example above, vertex C has an in-degree of 2 (edges coming from A and B), and vertex A has an outdegree of 2 (edges going to B and C).
3. Cycles
A cycle in a directed graph occurs when a path exists that starts and ends at the same vertex, with at least one edge being traversed. Cycles in directed graphs are crucial in various contexts such as detecting feedback loops or infinite loops in systems.
For example, in project management, cycles in task dependencies can indicate that tasks are dependent on each other in a circular manner, which is problematic as it leads to deadlocks. Similarly, in computer networks, cycles can help detect redundant pathways that may affect routing efficiency.
A directed graph without any cycles is called a Directed Acyclic Graph (DAG), which is used extensively in task scheduling algorithms, version control systems (e.g., Git), and dependency resolution.
// Example in C++ to check for cycles in a directed graph
#include <iostream>
#include <vector>
class DirectedGraph {
int V;
std::vector<std::vector<int>> adj;
bool isCyclicUtil(int v, std::vector<bool> &visited, std::vector<bool> &recStack);
public:
DirectedGraph(int V);
void addEdge(int v, int w);
bool isCyclic();
};
DirectedGraph::DirectedGraph(int V) {
this->V = V;
adj.resize(V);
}
void DirectedGraph::addEdge(int v, int w) {
adj[v].push_back(w);
}
bool DirectedGraph::isCyclicUtil(int v, std::vector<bool> &visited, std::vector<bool> &recStack) {
if (!visited[v]) {
visited[v] = true;
recStack[v] = true;
for (int neighbor : adj[v]) {
if (!visited[neighbor] && isCyclicUtil(neighbor, visited, recStack)) {
return true;
} else if (recStack[neighbor]) {
return true;
}
}
}
recStack[v] = false;
return false;
}
bool DirectedGraph::isCyclic() {
std::vector<bool> visited(V, false);
std::vector<bool> recStack(V, false);
for (int i = 0; i < V; i++) {
if (isCyclicUtil(i, visited, recStack)) {
return true;
}
}
return false;
}
int main() {
DirectedGraph g(4);
g.addEdge(0, 1);
g.addEdge(1, 2);
g.addEdge(2, 3);
g.addEdge(3, 1);
if (g.isCyclic())
std::cout << "Graph contains cycle\n";
else
std::cout << "Graph does not contain cycle\n";
return 0;
}
// Output is...
// Graph contains cycle
4. Paths and Reachability
In a directed graph, a path between two vertices follows the direction of the edges. Unlike in undirected graphs, where any edge can be traversed in either direction, in a directed graph, paths are restricted by the edge directions. This makes the concept of reachability—whether a vertex can be reached from another—especially important.
For example, in transportation networks, represented as directed graphs, a path from city A to city B exists only if there is a route connecting A to B, following the directed edges.
In software development, reachability is critical when analyzing call graphs (directed graphs representing function calls in programs). In static code analysis, we use directed graphs to check which functions or methods are reachable from a given starting point, helping detect dead code or unreachable code paths.
Applications of Directed Graphs
Directed graphs have a broad range of applications across different domains. Here are some notable examples:
1. Social Networks
Social networks can be modeled as directed graphs, where each person is a vertex, and edges represent relationships such as friendships, followers, or connections. For instance, Twitter can be modeled as a directed graph, where an edge from user A to user B means that A follows B, but B might not follow A back.
2. Transportation Networks
Transportation systems such as road networks, airline routes, or subway systems can be modeled as directed graphs. Each location (e.g., city or station) is a vertex, and each route between locations is a directed edge. In many cases, the edges are directed because not all routes are bidirectional. For instance, one-way streets in a city or flight routes between two cities (which may not have return flights) can be represented as directed edges.
3. Computer Networks
In computer networks, routers, switches, and devices form a directed graph, where each edge represents a communication link between devices. The directionality of edges in this context could represent data flow or communication protocols. Understanding directed graphs in this context helps in optimizing routing algorithms, such as Dijkstra’s shortest path algorithm and Bellman-Ford algorithm, which help in efficiently routing packets through the network.
4. Project Management
In project management, tasks, and their dependencies can be represented using directed graphs, where each task is a vertex and an edge from task A to task B signifies that task A must be completed before task B can begin. Tools like PERT (Program Evaluation Review Technique) and CPM (Critical Path Method) rely on this kind of structure to calculate the critical path—the longest sequence of dependent tasks that determines the project’s minimum completion time.
// Example in Java for representing a project using a Directed Acyclic Graph (DAG)
import java.util.*;
class TaskGraph {
private final Map<String, List<String>> adjList = new HashMap<>();
public void addTask(String task) {
adjList.putIfAbsent(task, new ArrayList<>());
}
public void addDependency(String fromTask, String toTask) {
adjList.get(fromTask).add(toTask);
}
public void printTasks() {
for (Map.Entry<String, List<String>> entry : adjList.entrySet()) {
System.out.print("Task " + entry.getKey() + " depends on: ");
for (String dependentTask : entry.getValue()) {
System.out.print(dependentTask + " ");
}
System.out.println();
}
}
}
public class ProjectManager {
public static void main(String[] args) {
TaskGraph taskGraph = new TaskGraph();
taskGraph.addTask("Design");
taskGraph.addTask("Development");
taskGraph.addTask("Testing");
taskGraph.addTask("Deployment");
taskGraph.addDependency("Design", "Development");
taskGraph.addDependency("Development", "Testing");
taskGraph.addDependency("Testing", "Deployment");
taskGraph.printTasks();
}
}
// Output is...
// Task Design depends on: Development
// Task Development depends on: Testing
// Task Deployment depends on:
// Task Testing depends on: Deployment
Advantages of Directed Graphs
- Model Complex Relationships: Directed graphs can represent complex relationships where directionality is critical, such as in social networks, transportation systems, or communication protocols.
- Dependency Representation: In tasks or workflows, directed graphs are excellent for representing dependencies, such as in project management or version control systems.
- Flow Analysis: Directed graphs can be used to analyze the flow of information or resources through a system, as in computer networks or business processes.
Disadvantages of Directed Graphs
- Increased Complexity: Directed graphs are often more complex to understand and analyze compared to undirected graphs. Each edge has directionality, and algorithms need to account for this, which can make analysis more computationally expensive.
- Less Intuitive: For those unfamiliar with directed graphs, they can be less intuitive to work with than undirected graphs, where the bidirectional nature of relationships simplifies understanding.
Conclusion
Directed graphs are a powerful tool for modeling a wide range of real-world problems where directionality is a fundamental aspect of relationships. From social networks to transportation systems and project management, directed graphs provide a structured way to represent and analyze complex systems. While they can introduce additional complexity, their ability to represent directed dependencies and flow makes them indispensable in fields ranging from computer science to engineering and management. By understanding the properties and applications of directed graphs, we can unlock new insights into the systems we rely on every day.
Related Articles
- Understanding Big-Theta (Θ) Notation in Algorithm Analysis
- Big-Omega (Ω) Notation in Algorithm Analysis: A Comprehensive Guide
- Big O Notation Tutorial – A Comprehensive Guide to Algorithm Complexity Analysis
- Asymptotic Notation and Complexity Analysis of Algorithms
- Understanding Algorithms in Computer Science: A Comprehensive Guide
- Understanding Trie Data Structure in Depth: A Comprehensive Guide
- Real-Life Example of the Brute Force Algorithm: Password Cracking
- Brute Force Algorithm: Comprehensive Exploration, Pros, Cons, & Applications
- Analyzing an Algorithm and its Complexity: A Comprehensive Guide
- Understanding Algorithms: A Comprehensive Introduction
- Understanding Hashing: The Key to Fast and Efficient Data Storage and Retrieval
- Hierarchical Data Structures: Binary Trees, Binary Search Trees, Heaps, & Hashing
- Comprehensive Overview on Applications of Arrays, Advantages & Disadvantages of Arrays
- Matrix Data Structure: A Comprehensive Guide to the Two-Dimensional Array
- Introduction to Array Data Structures: A Comprehensive Guide
- Understanding Linear Data Structures: A Comprehensive Exploration
- Difference Between Linear & Non-Linear Data Structures: A Comprehensive Overview
- Tree Data Structures: Definitions, Types, Applications, & Comprehensive Exploration
- Cyclic Graphs: Structure, Applications, Advantages, & Challenges in Data Structures
- Introduction to Directed Acyclic Graph (DAG): A Comprehensive Exploration with Examples
- Strongly, Unilaterally, and Weakly Connected Graphs in Data Structures
- Unweighted Graphs: Definition, Applications, Advantages, and Disadvantages
- Comprehensive Guide to Adjacency Lists in Data Structures
- Adjacency Matrix: A Comprehensive Guide to Graph Representation
- Understanding Weighted Graphs: A Comprehensive Exploration
- Understanding Undirected Graphs: Structure, Applications, and Advantages
- Understanding Directed Graphs: Characteristics, Applications, & Real-World Examples
- Graph Data Structure in Computer Science: A Comprehensive Exploration
- Understanding Data Structures: An In-Depth Exploration
- A Comprehensive Guide to DSA: Data Structures and Algorithms
Read More Articles
- Data Structure (DS) Array:
- Why the Analysis of Algorithms is Important?
- Worst, Average, and Best Case Analysis of Algorithms: A Comprehensive Guide
- Understanding Pointers in C Programming: A Comprehensive Guide
- Understanding Arrays in Data Structures: A Comprehensive Exploration
- Memory Allocation of an Array: An In-Depth Comprehensive Exploration
- Understanding Basic Operations in Arrays: A Comprehensive Guide
- Understanding 2D Arrays in Programming: A Comprehensive Guide
- Mapping a 2D Array to a 1D Array: A Comprehensive Exploration
- Data Structure Linked List:
- Understanding Linked Lists in Data Structures: A Comprehensive Exploration
- Types of Linked List: Detailed Exploration, Representations, and Implementations
- Understanding Singly Linked Lists: A Detailed Exploration
- Understanding Doubly Linked List: A Comprehensive Guide
- Operations of Doubly Linked List with Implementation: A Detailed Exploration
- Insertion in Doubly Linked List with Implementation: A Detailed Exploration
- Inserting a Node at the beginning of a Doubly Linked List: A Detailed Exploration
- Inserting a Node After a Given Node in a Doubly Linked List: A Detailed Exploration
- Inserting a Node Before a Given Node in a Doubly Linked List: A Detailed Exploration
- Inserting a Node at a Specific Position in a Doubly Linked List: A Detailed Exploration
- Inserting a New Node at the End of a Doubly Linked List: A Detailed Exploration
- Deletion in a Doubly Linked List with Implementation: A Comprehensive Guide
- Deletion at the Beginning in a Doubly Linked List: A Detailed Exploration
- Deletion after a given node in Doubly Linked List: A Comprehensive Guide
- Deleting a Node Before a Given Node in a Doubly Linked List: A Detailed Exploration
- Deletion at a Specific Position in a Doubly Linked List: A Detailed Exploration
- Deletion at the End in Doubly Linked List: A Comprehensive Exploration
- Introduction to Circular Linked Lists: A Comprehensive Guide
- Understanding Circular Singly Linked Lists: A Comprehensive Guide
- Circular Doubly Linked List: A Comprehensive Guide
- Insertion in Circular Singly Linked List: A Comprehensive Guide
- Insertion in an Empty Circular Linked List: A Detailed Exploration
- Insertion at the Beginning in Circular Linked List: A Detailed Exploration
- Insertion at the End of a Circular Linked List: A Comprehensive Guide
- Insertion at a Specific Position in a Circular Linked List: A Detailed Exploration
- Deletion from a Circular Linked List: A Comprehensive Guide
- Deletion from the Beginning of a Circular Linked List: A Detailed Exploration
- Deletion at Specific Position in Circular Linked List: A Detailed Exploration
- Deletion at the End of a Circular Linked List: A Comprehensive Guide
- Searching in a Circular Linked List: A Comprehensive Exploration
Frequently Asked Questions (FAQs) on Directed Graphs
What is a Directed Graph, and how does it differ from an Undirected Graph?
A directed graph, also known as a digraph, is a graph where each edge has a direction associated with it, indicating a one-way relationship between vertices. In contrast, in an undirected graph, edges have no direction, meaning the relationship between vertices is bidirectional.
In a directed graph, an edge from vertex A to vertex B means you can traverse from A to B, but not necessarily from B to A unless there’s an explicit edge from B to A. This concept is essential for modeling relationships where direction matters, such as in social networks (e.g., Twitter, where a user can follow another without reciprocation), transportation systems (e.g., one-way roads), or dependencies in projects.
For example, if you want to model a one-way street in a city, you’d use a directed edge to signify traffic can only flow in one direction. Here’s a Python example for representing a directed graph:
# Example of a directed graph in Python using adjacency lists
class DirectedGraph:
def __init__(self):
self.graph = {}
def add_edge(self, u, v):
if u in self.graph:
self.graph[u].append(v)
else:
self.graph[u] = [v]
def display(self):
for vertex in self.graph:
print(f"{vertex} -> {self.graph[vertex]}")
g = DirectedGraph()
g.add_edge('A', 'B')
g.add_edge('B', 'C')
g.add_edge('C', 'D')
g.add_edge('A', 'D')
g.display()
# Output is...
# A -> ['B', 'D']
# B -> ['C']
# C -> ['D']
In this example, the graph has directed edges such as A → B, B → C, etc. Traversal is allowed only in the direction specified by the edges.
What are the key characteristics of a Directed Graph?
A directed graph has several unique characteristics:
- Directed Edges: Each edge in a directed graph has a direction, forming a one-way connection between two vertices.
- Indegree and Outdegree: In a directed graph, each vertex has two measures of degree: indegree, which is the number of incoming edges to the vertex, and outdegree, which is the number of outgoing edges from the vertex.
- Cycles: A cycle occurs when a path in a directed graph starts and ends at the same vertex. In directed graphs, cycles can indicate feedback loops or circular dependencies.
- Acyclic Property: A Directed Acyclic Graph (DAG) is a type of directed graph that contains no cycles. DAGs are commonly used in task scheduling and dependency resolution.
- Paths and Reachability: In directed graphs, a path must follow the direction of the edges, and reachability is determined by whether a vertex can be reached by following the directed edges.
Here’s an example in C++ that demonstrates the concept of indegree and outdegree:
#include <iostream>
#include <vector>
class DirectedGraph {
int V;
std::vector<std::vector<int>> adj;
public:
DirectedGraph(int V);
void addEdge(int v, int w);
int indegree(int v);
int outdegree(int v);
};
DirectedGraph::DirectedGraph(int V) {
this->V = V;
adj.resize(V);
}
void DirectedGraph::addEdge(int v, int w) {
adj[v].push_back(w);
}
int DirectedGraph::indegree(int v) {
int count = 0;
for (const auto& neighbors : adj) {
for (int neighbor : neighbors) {
if (neighbor == v) {
count++;
}
}
}
return count;
}
int DirectedGraph::outdegree(int v) {
return adj[v].size();
}
int main() {
DirectedGraph g(4);
g.addEdge(0, 1);
g.addEdge(1, 2);
g.addEdge(2, 3);
g.addEdge(0, 3);
std::cout << "Indegree of vertex 3: " << g.indegree(3) << std::endl;
std::cout << "Outdegree of vertex 0: " << g.outdegree(0) << std::endl;
return 0;
}
# Output is...
# Indegree of vertex 3: 2
# Outdegree of vertex 0: 2
What is the significance of Indegree and Outdegree in Directed Graphs?
In a directed graph, each vertex has two key metrics related to connectivity: indegree and outdegree.
- Indegree: This is the number of incoming edges to a vertex. It represents how many other vertices have directed edges leading to this vertex. For example, in a social network where A follows B, B would have an in-degree of 1 (indicating one person follows them).
- Outdegree: This is the number of outgoing edges from a vertex. It represents how many vertices this vertex points to. In the same social network example, A would have an outdegree of 1, indicating they are following one person.
In real-world applications like task dependency graphs or computer networks, understanding the indegree and outdegree of vertices can help optimize workflow scheduling or network routing.
Here’s an example in Java:
import java.util.HashMap;
import java.util.List;
import java.util.ArrayList;
class DirectedGraph {
HashMap<String, List<String>> graph = new HashMap<>();
public void addEdge(String from, String to) {
graph.computeIfAbsent(from, k -> new ArrayList<>()).add(to);
}
public int indegree(String node) {
int indegreeCount = 0;
for (String key : graph.keySet()) {
if (graph.get(key).contains(node)) {
indegreeCount++;
}
}
return indegreeCount;
}
public int outdegree(String node) {
return graph.getOrDefault(node, new ArrayList<>()).size();
}
}
public class Main {
public static void main(String[] args) {
DirectedGraph graph = new DirectedGraph();
graph.addEdge("A", "B");
graph.addEdge("B", "C");
graph.addEdge("C", "D");
System.out.println("Indegree of C: " + graph.indegree("C"));
System.out.println("Outdegree of A: " + graph.outdegree("A"));
}
}
// Output is...
// Indegree of C: 1
// Outdegree of A: 1
What are Cycles in Directed Graphs, and how are they detected?
A cycle in a directed graph occurs when a path starts and ends at the same vertex, following the direction of the edges. Cycles are an essential concept in directed graphs, as they represent feedback loops or circular dependencies, which can lead to problems such as deadlock in systems.
For example, in project management, if Task A depends on Task B, and Task B depends on Task A, this creates a cycle, meaning neither task can be completed without the other, causing a deadlock.
Detecting cycles in directed graphs is crucial for many applications. In DAGs (Directed Acyclic Graphs), cycles are forbidden, as they represent tasks that cannot be scheduled.
Here’s an example in C++ for detecting cycles in a directed graph:
#include <iostream>
#include <vector>
class DirectedGraph {
int V;
std::vector<std::vector<int>> adj;
bool isCyclicUtil(int v, std::vector<bool> &visited, std::vector<bool> &recStack);
public:
DirectedGraph(int V);
void addEdge(int v, int w);
bool isCyclic();
};
DirectedGraph::DirectedGraph(int V) {
this->V = V;
adj.resize(V);
}
void DirectedGraph::addEdge(int v, int w) {
adj[v].push_back(w);
}
bool DirectedGraph::isCyclicUtil(int v, std::vector<bool> &visited, std::vector<bool> &recStack) {
if (!visited[v]) {
visited[v] = true;
recStack[v] = true;
for (int neighbor : adj[v]) {
if (!visited[neighbor] && isCyclicUtil(neighbor, visited, recStack)) {
return true;
} else if (recStack[neighbor]) {
return true;
}
}
}
recStack[v] = false;
return false;
}
bool DirectedGraph::isCyclic() {
std::vector<bool> visited(V, false);
std::vector<bool> recStack(V, false);
for (int i = 0; i < V; i++) {
if (isCyclicUtil(i, visited, recStack)) {
return true;
}
}
return false;
}
int main() {
DirectedGraph g(4);
g.addEdge(0, 1);
g.addEdge(1, 2);
g.addEdge(2, 0);
g.addEdge(2, 3);
if (g.isCyclic())
std::cout << "Graph contains a cycle\n";
else
std::cout << "Graph does not contain a cycle\n";
return 0;
}
# Output is...
# Graph contains a cycle
In this example, a DFS (Depth FirstSearch) approach is used to detect cycles by keeping track of visited nodes and the recursion stack.
What is a Directed Acyclic Graph (DAG)? How is it different from a regular Directed Graph?
A Directed Acyclic Graph (DAG) is a type of directed graph that contains no cycles, meaning there is no way to start at a vertex and return to it by following the directed edges. DAGs are widely used in situations where tasks must be ordered, as they can represent dependencies without leading to circular dependencies, which would cause a deadlock.
Some common uses of DAGs include:
- Task scheduling: In project management, where each task depends on one or more previous tasks, a DAG can represent the task hierarchy.
- Version control: Systems like Git use DAGs to represent changes and commits, ensuring no cyclical dependencies.
- Topological sorting: A technique used on DAGs to find an order in which tasks or events can be executed.
Here’s an example of topological sorting using Java:
import java.util.*;
class DAG {
private final int V;
private final List<List<Integer>> adj;
public DAG(int V) {
this.V = V;
adj = new ArrayList<>(V);
for (int i = 0; i < V; i++) {
adj.add(new ArrayList<>());
}
}
public void addEdge(int v, int w) {
adj.get(v).add(w);
}
private void topologicalSortUtil(int v, boolean[] visited, Stack<Integer> stack) {
visited[v] = true;
for (int i : adj.get(v)) {
if (!visited[i]) {
topologicalSortUtil(i, visited, stack);
}
}
stack.push(v);
}
public void topologicalSort() {
Stack<Integer> stack = new Stack<>();
boolean[] visited = new boolean[V];
for (int i = 0; i < V; i++) {
if (!visited[i]) {
topologicalSortUtil(i, visited, stack);
}
}
while (!stack.isEmpty()) {
System.out.print(stack.pop() + " ");
}
}
}
public class Main {
public static void main(String[] args) {
DAG g = new DAG(6);
g.addEdge(5, 2);
g.addEdge(5, 0);
g.addEdge(4, 0);
g.addEdge(4, 1);
g.addEdge(2, 3);
g.addEdge(3, 1);
System.out.println("Topological sorting of the DAG:");
g.topologicalSort();
}
}
// Output is...
// Topological sorting of the DAG:
// 5 4 2 3 1 0
In this Java example, the DAG represents tasks, and the topological sort provides an order in which tasks should be completed.
What is a Path in a Directed Graph, and how does Reachability work?
In a directed graph, a path is a sequence of vertices connected by directed edges, where you must follow the direction of the edges. Reachability refers to whether there exists a path between two vertices. If there is a path from vertex A to vertex B, then B is said to be reachable from A.
Understanding reachability is critical in many real-world scenarios, such as determining whether a website is accessible from another in computer networks or finding dependencies between tasks in project management.
For instance, if you’re using PHP to represent a directed graph, you can check for reachability as follows:
<?php
class DirectedGraph {
private $graph = array();
public function addEdge($u, $v) {
$this->graph[$u][] = $v;
}
public function isReachable($u, $v) {
if (!isset($this->graph[$u])) {
return false;
}
$visited = array();
return $this->dfs($u, $v, $visited);
}
private function dfs($u, $v, &$visited) {
if ($u == $v) {
return true;
}
$visited[$u] = true;
foreach ($this->graph[$u] as $neighbor) {
if (!isset($visited[$neighbor]) && $this->dfs($neighbor, $v, $visited)) {
return true;
}
}
return false;
}
}
$g = new DirectedGraph();
$g->addEdge("A", "B");
$g->addEdge("B", "C");
$g->addEdge("C", "D");
echo $g->isReachable("A", "D") ? "A can reach D" : "A cannot reach D";
?>
/* Output is... */
/* A can reach D */
In this PHP example, a simple DFS (Depth First Search) is used to check whether vertex A can reach vertex D by following the directed edges.
How do you represent Directed Graphs in Programming?
There are multiple ways to represent directed graphs in programming:
- Adjacency Matrix: This is a 2D array where each element represents whether there’s an edge between two vertices. An adjacency matrix is efficient for dense graphs but consumes a lot of memory.
- Adjacency List: This is a list where each vertex stores a list of its neighbors (vertices to which it has directed edges). It’s more memory efficient, especially for sparse graphs.
Here’s an example in Python using an Adjacency List:
class DirectedGraph:
def __init__(self):
self.graph = {}
def add_edge(self, u, v):
if u not in self.graph:
self.graph[u] = []
self.graph[u].append(v)
def display(self):
for vertex, neighbors in self.graph.items():
print(f"{vertex} -> {', '.join(neighbors)}")
g = DirectedGraph()
g.add_edge('A', 'B')
g.add_edge('A', 'C')
g.add_edge('B', 'D')
g.display()
In this Python example, the adjacency list allows you to store edges in a more compact form than a matrix.
How are Directed Graphs Used in Social Networks?
In social networks, directed graphs are commonly used to represent relationships where direction matters, such as on Twitter, where users can follow others without needing mutual consent. In this context, each user is represented as a vertex, and directed edges represent the “following” relationship.
For example:
- A → B means A follows B, but B does not necessarily follow A.
- Mutual follows can be represented by two edges: A → B and B → A.
Analyzing these directed edges can provide insights into influence (users with many followers) and reach (how far information can spread from one user to another).
Here’s how you can represent a social network graph in C++:
#include <iostream>
#include <vector>
#include <map>
class SocialNetworkGraph {
std::map<std::string, std::vector<std::string>> graph;
public:
void addUser(std::string user) {
if (graph.find(user) == graph.end()) {
graph[user] = std::vector<std::string>();
}
}
void addFollow(std::string follower, std::string followee) {
graph[follower].push_back(followee);
}
void displayNetwork() {
for (const auto& user : graph) {
std::cout << user.first << " follows: ";
for (const std::string& followee : user.second) {
std::cout << followee << " ";
}
std::cout << std::endl;
}
}
};
int main() {
SocialNetworkGraph graph;
graph.addUser("Alice");
graph.addUser("Bob");
graph.addUser("Charlie");
graph.addFollow("Alice", "Bob");
graph.addFollow("Alice", "Charlie");
graph.addFollow("Bob", "Charlie");
graph.displayNetwork();
return 0;
}
This simple C++ implementation shows how users follow others in a directed graph structure.
What is Topological Sorting in Directed Graphs, and where is it used?
Topological sorting is a linear ordering of vertices in a DAG where, for every directed edge u → v, vertex u appears before v in the ordering. It’s commonly used in:
- Task scheduling: When tasks depend on one another, topological sorting can be used to find an order in which tasks should be executed.
- Compilation: In programming languages, topological sorting can help resolve dependencies between files or modules.
Here’s how topological sorting can be implemented in C#:
using System;
using System.Collections.Generic;
class Graph {
private int V;
private List<int>[] adj;
public Graph(int V) {
this.V = V;
adj = new List<int>[V];
for (int i = 0; i < V; i++) {
adj[i] = new List<int>();
}
}
public void AddEdge(int v, int w) {
adj[v].Add(w);
}
public void TopologicalSortUtil(int v, bool[] visited,
Stack<int> stack) {
visited[v] = true;
foreach (int neighbor in adj[v]) {
if (!visited[neighbor]) {
TopologicalSortUtil(neighbor, visited, stack);
}
}
stack.Push(v);
}
public void TopologicalSort() {
Stack<int> stack = new Stack<int>();
bool[] visited = new bool[V];
for (int i = 0; i < V; i++) {
if (!visited[i]) {
TopologicalSortUtil(i, visited, stack);
}
}
while (stack.Count > 0) {
Console.Write(stack.Pop() + " ");
}
}
}
class Program {
static void Main(string[] args) {
Graph g = new Graph(6);
g.AddEdge(5, 2);
g.AddEdge(5, 0);
g.AddEdge(4, 0);
g.AddEdge(4, 1);
g.AddEdge(2, 3);
g.AddEdge(3, 1);
Console.WriteLine("Topological sort of the graph:");
g.TopologicalSort();
}
}
This C# code shows a simple example of topological sorting using a DAG.
What are the most common applications of Directed Graphs?
Directed graphs have applications across a broad spectrum of fields:
- Social networks: Representing following/friendship relationships.
- Transportation networks: Representing one-way roads or flight paths.
- Computer networks: Modeling directed connections between devices.
- Project management: Representing task dependencies and precedence constraints.
- Internet and web navigation: Websites linking to other websites are modeled using directed edges, where each website is a vertex.
- Recommender systems: Directed graphs can model relationships between users and items, where edges represent preferences or recommendations.
How are Directed Graphs used in task scheduling?
In task scheduling, a directed graph can represent tasks as vertices and dependencies between tasks as directed edges. If Task A must be completed before Task B, an edge A → B is created. This ensures that the order of execution follows the dependencies.
DAGs are typically used in task scheduling because they guarantee there are no circular dependencies, allowing for a topological sort of task.
For example, JavaScript can be used to visualize task scheduling:
class TaskScheduler {
constructor() {
this.graph = {};
}
addTask(task) {
if (!this.graph[task]) {
this.graph[task] = [];
}
}
addDependency(task1, task2) {
this.graph[task1].push(task2);
}
printSchedule() {
for (const task in this.graph) {
console.log(`${task} -> ${this.graph[task].join(", ")}`);
}
}
}
const scheduler = new TaskScheduler();
scheduler.addTask('Task 1');
scheduler.addTask('Task 2');
scheduler.addTask('Task 3');
scheduler.addDependency('Task 1', 'Task 2');
scheduler.addDependency('Task 2', 'Task 3');
scheduler.printSchedule();
What is the role of Directed Graphs in Recommender Systems?
In recommender systems, a directed graph can model relationships between users and items. Vertices represent users and items, while edges represent preferences or interactions (such as a user liking or purchasing an item). Directed edges can point from a user to an item, indicating a directed preference.
Recommender systems can use graph traversal algorithms to find similar users or items by exploring these directed edges.
For example, if User A likes Item X, and User B is similar to User A, the system can recommend Item X to User B by traversing the graph.
How do you traverse a Directed Graph?
There are two common traversal methods for a directed graph:
- Depth First Search (DFS): A recursive approach where you explore each vertex as deep as possible before backtracking.
- Breadth First Search (BFS): A level-by-level exploration of vertices using a queue.
In Python, DFS can be implemented as follows:
class DirectedGraph:
def __init__(self):
self.graph = {}
def add_edge(self, u, v):
if u not in self.graph:
self.graph[u] = []
self.graph[u].append(v)
def dfs(self, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start)
for neighbor in self.graph.get(start, []):
if neighbor not in visited:
self.dfs(neighbor, visited)
g = DirectedGraph()
g.add_edge('A', 'B')
g.add_edge('B', 'C')
g.add_edge('A', 'C')
g.dfs('A')
This Python code shows a simple DFS traversal, exploring all vertices connected by directed edges.
How are Directed Graphs Used in Computer Networks?
In computer networks, devices such as computers, routers, and switches can be represented as vertices, while directed edges represent connections that send data in a specific direction. Directed graphs are useful in analyzing routing protocols, determining data flow, and optimizing network bandwidth.
For example, in routing algorithms such as Dijkstra’s algorithm or Bellman-Ford’s algorithm, directed graphs are used to find the shortest path from one device to another, taking into account the directionality of data flow.
What are Strongly Connected Components (SCCs) in Directed Graphs?
A Strongly Connected Component (SCC) is a maximal subgraph of a directed graph where there is a path between any two vertices. This means that, within an SCC, every vertex can be reached from every other vertex.
SCCs are useful in identifying clusters of vertices in social networks, where all members are highly interconnected. SCC detection is often performed using Kosaraju’s algorithm or Tarjan’s algorithm.
How do you detect Strongly Connected Components in a Directed Graph?
One way to detect SCCs is using Kosaraju’s algorithm, which involves two passes of DFS. The first pass identifies the finish times of each vertex, and the second pass, on the transposed graph, groups vertices into SCCs.
Here’s a simplified example in Python:
class DirectedGraph:
def __init__(self):
self.graph = {}
self.transpose_graph = {}
def add_edge(self, u, v):
self.graph.setdefault(u, []).append(v)
self.transpose_graph.setdefault(v, []).append(u)
def dfs(self, v, visited, stack=None):
visited.add(v)
if stack is not None:
stack.append(v)
for neighbor in self.graph.get(v, []):
if neighbor not in visited:
self.dfs(neighbor, visited, stack)
def dfs_transpose(self, v, visited):
visited.add(v)
print(v, end=" ")
for neighbor in self.transpose_graph.get(v, []):
if neighbor not in visited:
self.dfs_transpose(neighbor, visited)
def find_sccs(self):
visited = set()
stack = []
for vertex in self.graph:
if vertex not in visited:
self.dfs(vertex, visited, stack)
visited.clear()
while stack:
vertex = stack.pop()
if vertex not in visited:
self.dfs_transpose(vertex, visited)
print()
g = DirectedGraph()
g.add_edge('A', 'B')
g.add_edge('B', 'C')
g.add_edge('C', 'A')
g.add_edge('B', 'D')
g.find_sccs()
This Python code implements Kosaraju’s algorithm to detect SCCs in a directed graph.
What are some real-world examples of Directed Acyclic Graphs (DAGs)?
DAGs are used in:
- Project management: Representing tasks and their dependencies to ensure tasks are executed in the correct order.
- Version control: Systems like Git use DAGs to represent commit histories.
- Workflow systems: DAGs ensure that jobs are executed in the right sequence in data pipelines.
How do Directed Graphs apply to the PageRank Algorithm?
PageRank is a ranking algorithm used by Google to rank websites. The web is modeled as a directed graph, where each website is a vertex, and links between websites are directed edges. The algorithm assigns ranks to vertices based on the number and quality of incoming edges.
The PageRank algorithm relies on graph traversal and probability theory to compute the rank of each webpage and is a key example of how directed graphs can be used to solve real-world problems in search engine optimization.
How are Directed Graphs Used in Compiler Design?
In compiler design, directed graphs are used to represent control flow graphs (CFGs), where vertices represent instructions or blocks of code, and directed edges represent the flow of control. Directed graphs are also used in dependency resolution for determining the order in which modules or files must be compiled.
What are some challenges in working with Directed Graphs?
Some challenges include:
- Cycle detection: Ensuring that no cyclic dependencies exist, especially in scheduling or dependency resolution tasks.
- Scalability: Large directed graphs, such as those representing social networks or web links, can become difficult to process efficiently.
- SCC identification: Detecting strongly connected components in large graphs can be computationally intensive.